The print and println methods in Java
The print() and println() methods are Java’s predefined methods used to display text output on the console. These methods are part of the Java API.
nanadwumor
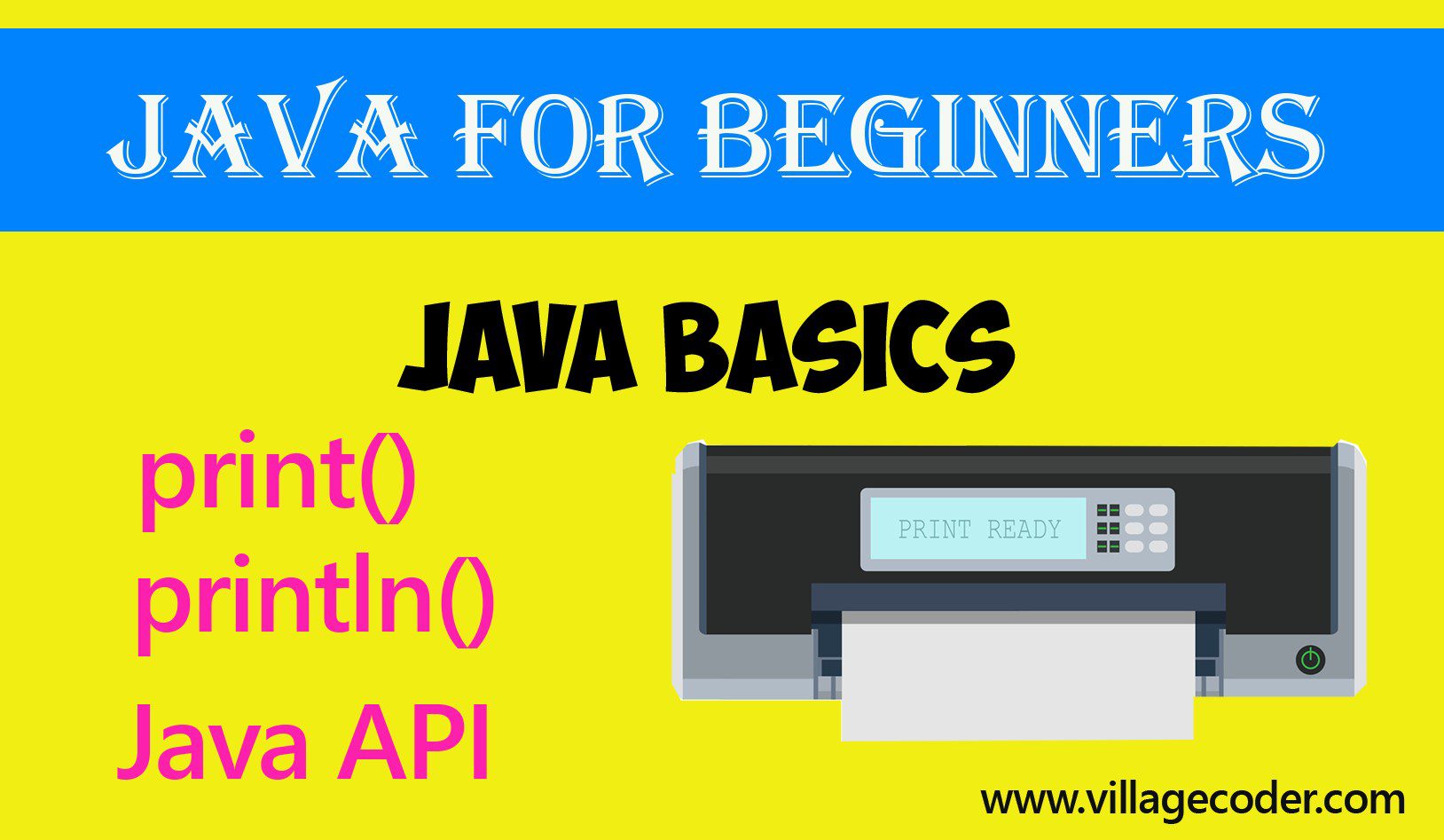
- The print and println methods are used to print string of characters onto the console
- The print method prints and leaves cursor on the same line
- The println method prints and moves cursor to the next line
RECOMMENDED ARTICLES
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures. In Java, a variable is a container used to store...
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal number. The double data type is a floating point number. It is double precision data type The range is 4.9406564584124654 x...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point number of 7 digits of accuracy. The float data type is a floating point number. It has single precision data type The float type is stored in 32 bits of...
The print() and println() methods are Java’s predefined methods used to display text output on the console. These methods are part of the Java API.
The term, API, stands for Application Programming Interface. The Java API is a collection of prewritten classes and methods for performing specific operations. These classes and methods are available to the programmer when coding his application.
Join Other Subscribers on Our YouTube Channel and Don’t Miss a thing!
Example of usage of print() method
System.out.print(“Thanks for visiting www.villagecoder.com”);
Explanation of above statement
The above statement displays the text Thanks for visiting www.villagecoder.com
Parameters the print() method accepts
The print() method accepts any parameter the programmer may wish to print out on the output screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
//This program demonstrates the print method public class PrintDemo { public static void main(String[] args) { System.out.print("Thank "); System.out.print("you "); System.out.print("for learning at "); System.out.print("villagecoder "); System.out.print("always."); } } |
Output


System : System is a class. It is found in java.lang.package. A class in Java contains methods and fields. A clas is used to construct objects in Java. Note that Java is an object oriented programming language.
That’s, to code in java, you create objects. And these objects are created from classes. So you see, classes are some sort of blueprint or plan from which you create your items or objects. This is similar to how a building constructor uses his building plan (the class) on paper to actually build a house (the object)
Where from the System class?
The System class is part of the Java API. This implies, you can call it anytime in your program if needed. Classes in the java.lang package are readily available to a program. System is a class that performs operations on system level.
Fields in System class
As said earlier, a class in Java has fields and methods (or functions). The fields store important information about the class (or objects created from the class). One example of the fields of the System class is out.
what is the ‘out’ field?
Mostly, fields are primitive values. Example of primitive values are int (for integer), float (for floating point number), double (another floating point number), boolean (true or false values) etc. However, a field in Java can also be an object. Example is a String.
String nameOfWebsite=“villagecoder”;
In the above statement, the field is called nameOfWebsite. It’s a String variable. Strings are objects but not primitive values. This implies, Strings have fields and methods on their own. Strings are enclosed in double quotation marks.
So you see, a field (which is an object) has fields and methods too. Don’t be confused! In simple terms, if the field of a class is an object (eg. a String), then it has its own fields and methods you can call.
String nameOfWebsite=“villagecoder”;
In the above statement, the String field is initialized to the value villagecoder. Because it’s a String, we can call one of its methods to perform operation on the value villagecoder.
Example, the statement nameOfWebsite.charAt(5) will print out g because g is the 5th character in the String villagecoder. charAt() is a pre-built method of the String class. Note that in Java, counting begins from 0, not 1 so g falls at the 5th position.
Now, is the ‘out’ field primitive or an object?
out is an object. This means out itself was created from another class. This class is called PrintStream class. This might sound confusing at a glance. The practice of creating an object from one class and using the object as a field in another class is called aggregation in Java.
out is public and static. public means it can be accessed by code outside the class. out is static means it belongs to the class but not instance of the class.
This implies that the class System can call its static field, out, straightaway without the need to create an instance (object) of System before calling the field, out. That is why we write:
System.out without creating any instance of the System class.
Methods of the out object
We have already established that, in Java, objects have both fields and methods which they can call when necessary. One of the methods of out (which serves as a field for System class) is the print() method.
Putting it all together
So why don’t programmers type just System.print() but System.out.print()? In other words, why wasn’t the print() method made a method of the System class but rather made a method of another class called PrintStream?
The System class has a number of functions including taking inputs and printing out data. Putting all these functions or methods under System would make it quite cumbersome.
Due to that, the brilliant designers of the Java language decided to group methods that perform similar functions under one umbrella and give them name.
Example, System methods that print out data are grouped together and given a class name PrintStream. To use PrintStream class, an object has to be created. One example of a PrintStream object is out, which is already created. Within the PrintStream class, we have methods such as print, println, printf, etc. Thus, the out object can call all these methods.
System.out.print()
System.out.println()
System.out.printf()
System functions that accept inputs from outside sources are grouped together and given a class name called InputStream. One object of InputStream is called in which is a field of the System class, written as, System.in.
The print() method
The print method prints string on the console and keeps cursor on the same line.
The print method is found in the out object.
After printing, the print() method leaves the cursor on the same line. Hence, subsequent printing instructions will print on the same line. Let’s look at example
1 2 3 4 5 6 7 8 9 10 11 |
//This program demonstrates that the print method //leaves cursor on the same line after executing public class PrintDemo { public static void main(String[] args) { System.out.print("The print method "); System.out.print("leaves cursor on the same line."); } } |
Output


The print method was called twice, one after the other. The first print method prints the message The print method and the cursor stays on the same line.
The second print method continues from where the cursor was left off. The cursor was left on the same line as the first statement. The second message leaves the cursor on the same line is printed in addition. The two messages appear on the same line in the output even though they were typed on different lines.
That is, print method displays text or message in a continuous stream.
Common errors associated with the print() method
Because the print method prints text and leaves the cursor on the same line, the displayed texts can be jammed up if space character is not left at the end of statements.
Let’s look at an example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
//This program demonstrates that the print method //jams strings of characters together if space character //is not inserted at end or beginning of the string public class NoSpace { public static void main(String[] args) { System.out.print("The print method"); System.out.print("leaves the cursor"); System.out.print("on the same"); System.out.print("line."); } } |
Ouput


The output is displayed in one string although the code uses four print() methods to display the message on each line. This is because each print method leaves the cursor on the same line after displaying text. This jams the strings on the different lines up. There are no spaces left in between the different lines of strings.
To remedy this we can type space character at the end of the preceding statement or at the beginning of the succeeding one.
Let’s look at how this can be done.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
//This program demonstrates how space character problem with the print method //can be solved public class NoSpaceRemedy { public static void main(String[] args) { System.out.print("The print method ");//space left at end of statement System.out.print("leaves the cursor");//no space character in this line. This is because space character is typed at beginning of next statement System.out.print(" on the same ");//space character typed at beginning and end of string System.out.print("line."); //No space character in string because one precedes the previous string. } } |
Ouput


How to make print() method print on different lines
The print method displays text and keeps the cursor on the same line. There’s a way to force the cursor to the next line even if you use the print method.
We type an escape character at the end of the string in order to force the cursor to the next line. An escape character begins with a backslash (\) and followed by a control sequence. There are different types of escape sequences. The escape sequence to move the cursor to next line is \n. n signifies newline.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
//This program demonstrates how to move the cursor //to next line using the newline escape sequence public class PrintNextLine { public static void main(String[] args) { System.out.print("The print method\n"); System.out.print("leaves the cursor\n"); System.out.print("on the same\n"); System.out.print("line."); } } |
Output
The print method
leaves the cursor
on the same
line.
The first print method displays The print method and gets to the escape sequence \n. This moves the cursor to the next line.
Note that the \n escape sequence is not printed by the print method. The print method interprets it as a special command and advances the cursor to the next line.
Escape Sequence
As the name suggests, an escape sequence enables the cursor to escape sequential movement of its path. An escape sequence is also called an escape character.
An escape sequence begins with a backslash (\) not a forward slash. That’s, don’t type /n but type \n.
Also, there shouldn’t be any space left between the backslash (\) and the character. That’s, don’t type \ n but \n.
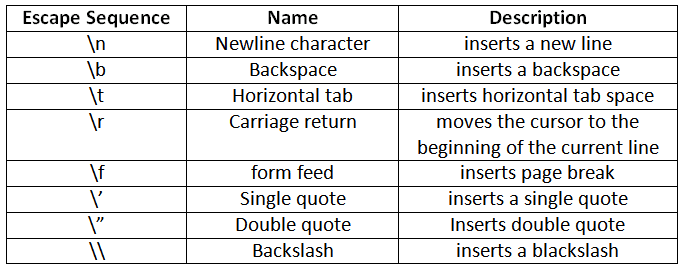
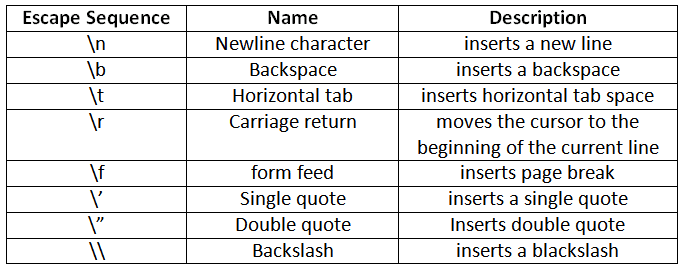
The print() method and data types
The text passed to the print method is normally a String. A String is an object and an object is a type of data in Java. There are other simple data types we call primitive data types. Examples of primitive data types in Java are int, double, float, short, byte, long, Boolean etc.
The print method can accept these data types and print on the console.
void print(boolean b) – Prints a boolean value.
Example
boolean status=true;
System.out.print(status);
Output
true
void print(char c) – Prints a character.
Example
char grade=’B’;
System.out.print(grade);
Output
B
void print(char[ ] s) – Prints an array of characters. .
Example
char[ ] grade={ ‘A‘,’B‘,’C’,’D‘,’E‘,’F‘};
System.out.print(grade);
Output
[A B C D E F]
void print(double d) –
Prints a double-precision floating-point number.
Example
double cost=34.7;
System.out.print(cost);
Output
34.5
void print(float f) –Prints a single-precision floating-point number.
Example
float cost=34.7f;
System.out.print(cost);
Output
34.7
void print(int i) –Prints an integer.
Example
int age=28;
System.out.print(age);
Output
28
void print(long l) –Prints a long integer.
Example
long age=28L;
System.out.print(age);
Output
28
void print(Object obj) –Prints an object.
Example
Object website==”www.villagecoder.com”;
System.out.print(website);
Output
www.villagecoder.com
void print(String s) –Prints a string.
Example
String website==”www.villagecoder.com”;
System.out.print(website);
Output
www.villagecoder.com
CAUTION : The print() method always needs arguments in order to execute. If arguments aren’t passed to it, it will cause an error.
System.out.print(); //error.No argument
Let’s look at a program that demonstrates that the print method creates an error without argument.
1 2 3 4 5 6 7 8 9 10 11 |
//This program demonstrates that //the print method always need argument to execute public class PrintWithNoArgument { public static void main(String[] args) { System.out.print();// Error : print method needs argument } } |
Output
1 2 3 4 |
Exception in thread "main" java.lang.Error: Unresolved compilation problem: The method print(boolean) in the type PrintStream is not applicable for the arguments () at javaBasics.PrintWithNoArgument.main(PrintWithNoArgument.java:11) |
The println() method
The println method displays text on the console just like the print method. However, the println method displays or prints text on the console and then moves the cursor to the beginning of the next line.
println is short for ‘print line’. That’s, println means print and move to next line.
Like the print method, the println method also accepts several data types. Let’s look at these data types :
void println() – Terminates the current line by writing the line separator string.
void println(boolean x) – Prints a boolean and then terminates the line.
void println(char x) – Prints a character variable and then terminates the line.
void println(char[] x) – Prints an array of characters and then terminate the line.
void println(double x) – Prints a double variable and then terminates the line.
void println(float x) – Prints a float variable and then terminates the line.
void println(int x) – Prints an integer variable and then terminates the line.
void println(long x) – Prints a long variable and then terminates the line.
void println(Object x) – Prints an Object and then terminates the line.
void println(String x) – Prints a String variable and then terminates the line.
Program demonstrates the println() method
1 2 3 4 5 6 7 8 9 10 11 12 13 |
//This program demonstrates the //println method public class PrintlnDemo { public static void main(String[] args) { System.out.println("This is the list of items:"); System.out.println("Sugar"); System.out.println("Bread"); System.out.println("Chocolate"); } } |
Output
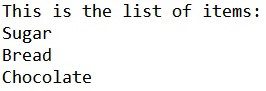
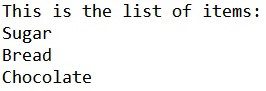
The println method prints or displays text and moves to the next line. We see that each invocation of the println method displays its text on a new line. Four println methods were called and statements were displayed on four different lines.
The first println displays This is the list of items: and then moves the cursor to the next line. The second println displays Sugar and moves the cursor to the next line. The third println method displays Bread and then moves the cursor to the next line. The fourth println method displays Chocolate and moves cursor to next line.
Using the println method to print empty spaces
The println method can execute without argument. This makes it possible to use it to print spaces between lines of code in order to make the code neat.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
//This program demonstrates that the println method //can be used to print whitespaces when it does //not take any argument public class SpacedCode { public static void main(String[] args) { System.out.println("This is statement One"); System.out.println(); //leave a space System.out.println("This is statement Two"); System.out.println();//leave a space System.out.println("This is statement Three"); System.out.println(); //leave a space System.out.println(); //leave a space System.out.println();//leave a space System.out.println("This is statement Four"); System.out.println("This is statement Five"); System.out.println("This is statement Six"); } } |
Output
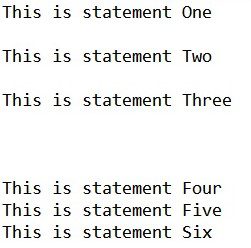
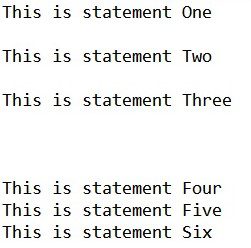
We called the println method to display statements on the console. The first statement is :
System.out.println(“This is statement One”); This displays This is statement One
After displaying this message, the cursor moves to the next line. Here we call the println method again but we do not pass any argument to it. Thus, it prints an empty space and moves to the third line.
We call the println method again and pass the statement “This is statement Two“. This gets printed and the cursor advances to the next line.
We call the println method again yet does not pass it any argument. This prints an empty space and the cursor moves to next line.
We call the println method again and pass the statement “This is statement Three“. This gets printed and the cursor advances to the next line.
Here, we call the println method three times in succession without passing any argument to it. The reason is to create three empty lines of space.
System.out.println();. //leave a space
System.out.println(); //leave a space
System.out.println();//leave a space
We want to separate the statements above from the next group of statements.
We call the println statements again this time passing arguments to it in each case.
System.out.println(“This is statement Four”);.
System.out.println(“This is statement Five”);
System.out.println(“This is statement Six”);
Because we do not print any space in between these statements, we see the statements stacked to each other.
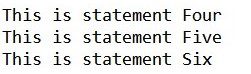
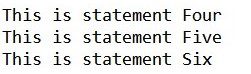
Differences between print and println method
We summarize the differences between the print and println method.
print() | println() |
Prints text and remains on same line | prints text and moves to the next line |
It always needs argument to execute else error will occur | It can execute without argument. In such casse, it prints empty line of space. |
You May Also Like…
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
double data type in Java
The double data type is also a floating point number. It is called double because it has a...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point...
0 Comments