How to take Strings apart with substring
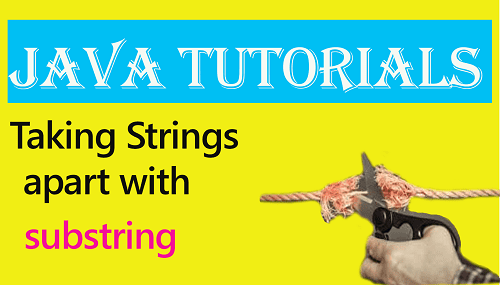
String is immutable. That is, you cannot change it. You can create smaller strings or substrings from a string. Whenever you create a substring, a new string is created in memory. The substring method is used to create a substring out of a string. A string can be broken into substrings by position using the substring method.
substring() method variants
The substring method has two overloaded forms. Each of these forms requires a starting index.
(1) substring(int beginIndex)
1 |
public String substring(int beginIndex) |
This method returns a substring of this string. That is, it returns a substring of the string that calls it. The substring starts from the character located at the specified index and extends to the end of the string. Note that the beginIndex is inclusive. That is, first character located at the beginIndex is included in the substring.
Example 1:
1 2 3 4 5 |
class SubstringDemo { public static void main(String[] args){ String name="Douglas"; //initialize a string String sub=name.substring(3); //substring starts from 'g' to the last character. Note that 'g' is at index 3 System.out.println(sub); //prints glas }} |
Output
1 |
glas |
The first character ‘D’ is at index 0, the second character ‘o’ is at index 1; the third character ‘u’ is at index 2; the fourth character ‘g’ is at index 3 and so on. Thus, substring(3) starts from the fourth character which is at index 3 up to the last character.
Example 2:
1 2 3 |
class SubstringDemo2 { public static void main(String[] args){ System.out.println("Douglas".substring(2)); //prints uglas |
Output
1 |
uglas |
The first character ‘D’ is at index 0, the second character ‘o’ is at index 1; the third character ‘u’ is at index 2 and so on. Thus, substring(2) starts from the third character ‘u’ which is located at index 2 up to the last character.
(2) substring(int beginIndex, int endIndex)
1 |
public String substring(int beginIndex, int endIndex) |
This form of the substring() method takes two arguments. The method returns a new string which is a substring of the this string. That is, it returns a substring of the string that calls it. The substring starts at the index beginIndex and ends at the endIndex-1. That is, 1 is subtracted from the value of the endIndex and the character at that point is returned as the last character. Example: If you write substring(1, 5) , the method will return the substring starting at index 1 but ending at index 4. That is, 1 is subtracted from the last index. Therefore, we can say that whereas the beginIndex is inclusive(included), the endIndex is exclusive (not included) when creating the substring.
Example 1:
1 2 3 4 5 6 7 |
class SubstringDemo { public static void main(String[] args){ String name="Douglas"; //initialize a string String sub=name.substring(2,7); //substring starts from 'u' to the (7-1) character. Note that the last index is exclusive3 System.out.println(sub); //prints uglas } } |
Output
1 |
uglas |
The string “Douglas” has 6 characters.
‘D’ is at index 0
‘o’ is at index 1
‘u’ is at index 2
‘g’ is at index 3
‘l’ is at index 4
‘a’ is at index 5
‘s’ is at index 6
This means substring(2,7) should have thrown an indexOutOfBoundsException because the last index is at 6, not 7. But because the substring() method’s last index is always minus 1, that is endIndex-1, the substring() method returns the substring starting from 2 alright but to (7-1), that is 6. But not 7. This mostly confusing too many beginners.
Recent Comments