How to use the switch statement in Java
The switch statement is a multi-way decision statement. It provides an easy way to change the path of execution to different parts of the program based on the value of the expression.
nanadwumor
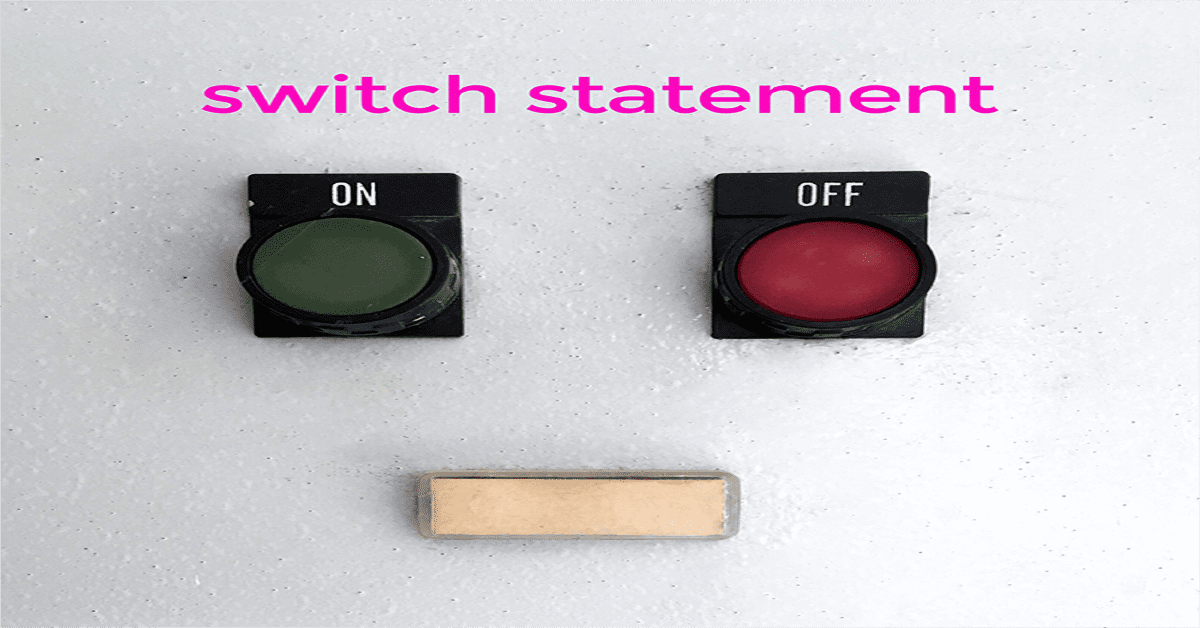
The expression passed to the switch statement can be a byte, short, char, int , enum data type.
Beginning with JDK7, switch was allowed to accept variables of String data types.
Hence, a switch statement accepts arguments of types : byte, short, int, char, enum, String.
Syntax of the switch statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
switch(textExpression) { case value1: //statement //statement break; case value2: //statement //statement break; case value2: //statement //statement break; . . . case valueN: //statement //statement break; default: //statement //statement } |
Join other Subscribers on our YouTube channel and enjoy daily pogramming tutorials.
How does the switch statement work?
The first line of the statement starts with the word switch which is followed by a testExpression. The testExpression is enclosed in parentheses.
The testExpression is of the data types char, byte, short, int, or string.
After the switch textExpression, a curly bracket opens to enclose all the internal code of the switch.
Inside the curly brackets are cases of the switch statement.
A case is simply one of the options the switch statement will select per the value of the testExpression.
A case section begins with the keyword case, followed by a value and a colon. This value will be tested against the testExpression and if they’re equal, the switch statement branches and gives control to that part of the code.
For each case, there are one or more statements which are followed by a break statement.
The break keyword ensures that after all statements under one case section are executed, control doesn’t continue to the next succeeding case. It literally “breaks” that path of execution.
After all of the case sections, the last section is an optional default section. This is the default section that will only execute if a case is not found to match the testExpression.
Also, the default statement will get executed even if a case is found in situations where proper break statements are not inserted.
Recommended
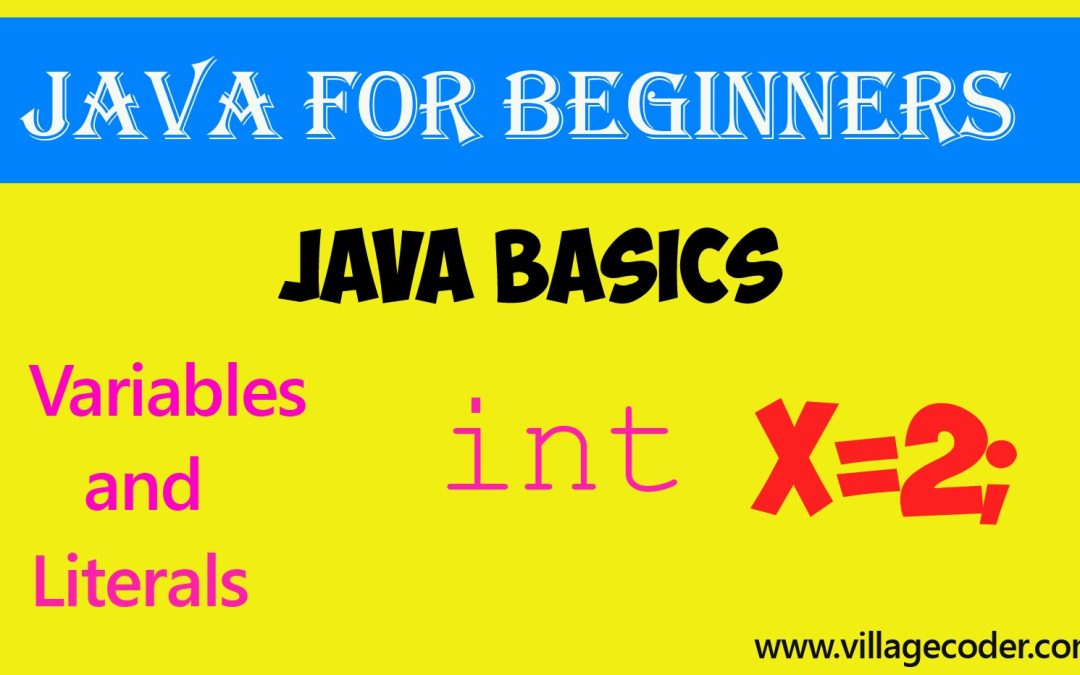
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
Program to demonstrate use of switch statement to print months of the year
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
import java.util.Scanner; //needed for the Scanner class /** * This program selects the months of the year based on int values from 1 * to 12 * * */ public class SwitchDemo { public static void main(String[] args) { int month; //to take input from the keyboard //Create a Scanner object Scanner in=new Scanner(System.in); //Get an input from the user System.out.print("Enter a number to see the corresponding month: "); month=in.nextInt(); switch(month) { case 1: System.out.println("January"); break; case 2: System.out.println("February"); break; case 3: System.out.println("March"); break; case 4: System.out.println("April"); break; case 5: System.out.println("May"); break; case 6: System.out.println("June"); break; case 7: System.out.println("July"); break; case 8: System.out.println("August"); break; case 9: System.out.println("September"); break; case 10: System.out.println("October"); break; case 11: System.out.println("November"); break; case 12: System.out.println("December"); break; default: System.out.println("Your number is INCORRECT. Select a number from 1-12"); break; } } } |
Output
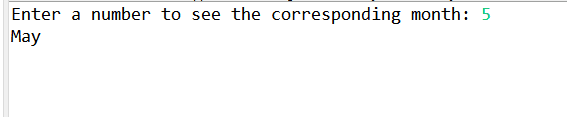
Explanation of code
If the value in the month variable is 1, the program will branch to the case 1 section and execute the statements under the case 1.
That’s, the statement System.out.print( “January”) will get executed.
If the value in the month variable is 2, the program will branch to the case 2 section and executes the statements at that case section.
That’s, the statement System.out.print(“February”) gets executed.
The break statement that comes after the statement causes the program to break. This causes the program to exit the switch statement.
If the value in the month variable is 3, the program will branch to the case 3 section and executes the statements at that case section.
That’s, the statement System.out.print(“March”) gets executed.
The break statement that comes after the statement causes the program to break. This causes the program to exit the switch statement.
In the same order, case 4 will print April; case 5 will print May with case 12 printing December.
If any number aside 1 to 12 is passed to the month variable, no case is selected so the program goes to the default statement and execute the statements there.
Flowchart of the switch statement
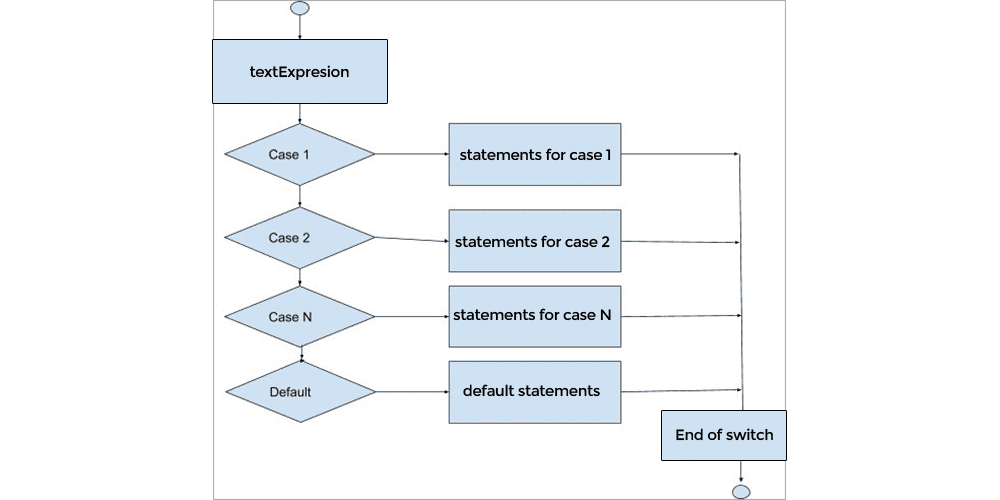
What rules govern the use of the switch statement?
- Duplicate cases are not allowed. That is, you cannot have the same case more than once in a switch statement. Example, the following code is a section of the program above. It takes an int value and prints out the corresponding month of the int value. The code has two cases with the value 2. This is wrong.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
switch(month) { case 1: System.out.println("January"); break; case 2: //This is wrong. A case cannot be repeated System.out.println("February"); break; case 2: //This is wrong. A case cannot be repeated System.out.println("March"); break; case 3: System.out.println("April"); break; default: System.out.println("Your number is INCORRECT. Select a number from 1-12"); break; } |
2. The value of the switch expression should be of the same data type as the value of the switch case. That is, if switch accepts int as textExpression, then the cases must also be int. In the same vein, if the texEpression is a String, the cases must have String values.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
public class SwitchDemo { public static void main(String[] args) { String textExpression="March"; //text expression is a String switch(textExpression) { case "January": //case is a also a String System.out.println("Janvier"); break; case "February": //case is a also a String System.out.println("Fevier"); break; case "March": //case is a also a String System.out.println("Mars"); break; case "April": //case is a also a String System.out.println("Avril"); break; default: System.out.println("Sorry, your choice is wrong."); break; } } |
- The value of a switch case must be a constant and not a variable. If a variable is used, it should be initialized to a constant literal.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
switch(3) { //The textExpression of the switch statement is 3, a constant case 1: System.out.println("This is number 1"); break; case 2: System.out.println("This is number 2"); break; case 3: System.out.println("This is number 3"); break; default: System.out.println("Sorry, selelct another choice."); break; } |
In this example, the switch expression is a variable initialized to a String literal.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
String textExpression="March"; //text expression is a variable initialized to a String literal switch(textExpression) { //text expression is a variable initialized to a String literal case "January": System.out.println("This month is January"); break; case "February": System.out.println("This month is February"); break; case "March": System.out.println("This month is March"); break; |
- The break statement is optional. It is used inside the switch to terminate a statement sequence. If omitted, execution will continue on into the next case. In the below switch statement, case ‘a’ and case ‘A’ do not have a break statement. This means, if the switch textExpression is ‘a’ , it will fall through to case ‘A’. The same is true for the other cases.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
int letter='c'; //to hold a letter choice switch(letter) { //text expression is a variable initialized to a char case 'a': //no break statement after this case case 'A': System.out.println("You entered letter a or A"); break; case 'b': //no break statement after this case case 'B': System.out.println("You entered letter b or B"); break; case 'c': //no break statement after this case case 'C': System.out.println("You entered letter c or C"); break; case 'd': //no break statement after this case case 'D': System.out.println("You entered letter d or D"); break; default: System.out.println("You didn't enter any RECOGNIZED letter"); break; } |
- The default statement is optional. The default statement is executed if none of the cases is selected and executed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
int letter='c'; //to hold a letter choice switch(letter) { //text expression is a variable initialized to a char case 'a': System.out.println("You entered letter a"); break; case 'b': System.out.println("You entered letter b"); break; case 'c': System.out.println("You entered letter c"); break; case 'd': System.out.println("You entered letter d"); break; } |
The switch statement and the if-else-if statement
The switch statement provides a relatively better alternative to a large series of if-else-if statement. The if-else-if statement becomes more complex if the options are many.
if-else-if program to calculate grade of student
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
import java.util.Scanner; //needed for the Scanner class public class IfElseDemo { public static void main(String[] args) { int month; //to hold the score of a student //create a Scanner object to hold input from the keyboard Scanner in=new Scanner(System.in); //Enter the mark of a student System.out.print("Enter number of month: "); month=in.nextInt(); //check grade of student if(month==1) { System.out.println("January"); } else if (month==2) { System.out.println("February"); } else if (month==3) { System.out.println("March"); } else if (month==4) { System.out.println("April"); } else if (month==5) { System.out.println("May"); } } } |
Equivalent switch program to calculate grade of student
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
import java.util.Scanner; //needed for the Scanner class /** * This program calculates the grade of a *student based on marks obtained * * */ public class SwitchDemo { public static void main(String[] args) { int month; //to hold the score of a student //create a Scanner object to hold input from the keyboard Scanner in=new Scanner(System.in); //Enter the mark of a student System.out.print("Enter number of month: "); month=in.nextInt(); switch(month) { //text expression is a variable initialized to a char case 1: System.out.println("January"); break; case 2: System.out.println("February"); break; case 3: System.out.println("March"); break; case 4: System.out.println("April"); break; case 5: System.out.println("may"); break; } } } |
switch statement with no breaks
In a switch statement, the break statement is optional. If you omit the break, execution will continue on into the next case.
It is sometimes desirable to have multiple cases without break statements between them. For example, consider the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
import java.util.Scanner; //needed for the Scanner class public class NoBreakStatment { public static void main(String[] args) { String month; //to hold input for a month //create a Scanner object Scanner in=new Scanner(System.in); //Enter a month System.out.print("Enter a month: "); month=in.nextLine().toLowerCase(); //take input String and convert all to lowercase switch(month) { case "june": //no break. Execution fall through to next case case "july": //no break. Execution fall through to next case case "august": System.out.println("It's SUMMER"); break; case "january": //no break. Execution fall through to next case case "february": //no break. Execution fall through to next case case "december": System.out.println("It's WINTER"); break; case "march": //no break. Execution fall through to next case case "april": //no break. Execution fall through to next case case "may": System.out.println("It's SPRING"); break; case "september": //no break. Execution fall through to next case case "october": //no break. Execution fall through to next case case "november": System.out.println("It's AUTUMN"); break; default: System.out.println("Type month correctly" ); } } } |
Output
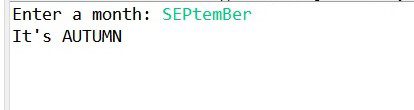
The default section is optional
Unless you want a default statement to execute if no case is selected, the default statement is optional and can be omitted just like in this example.
The break statement at the default section is optional
When the program gets to the default section, it executes the statements under that section and comes out of the switch statement even if there is no break statement.
This is because the default section is the last section. You may decide to put a break there or not. The program will still halt after execution.
You May Also Like…
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point number of 7 digits of...
Recent Comments