if statement in Java
An if statement in Java is a one way conditional statement that decides the execution path based on whether the condition is true or false.
nanadwumor
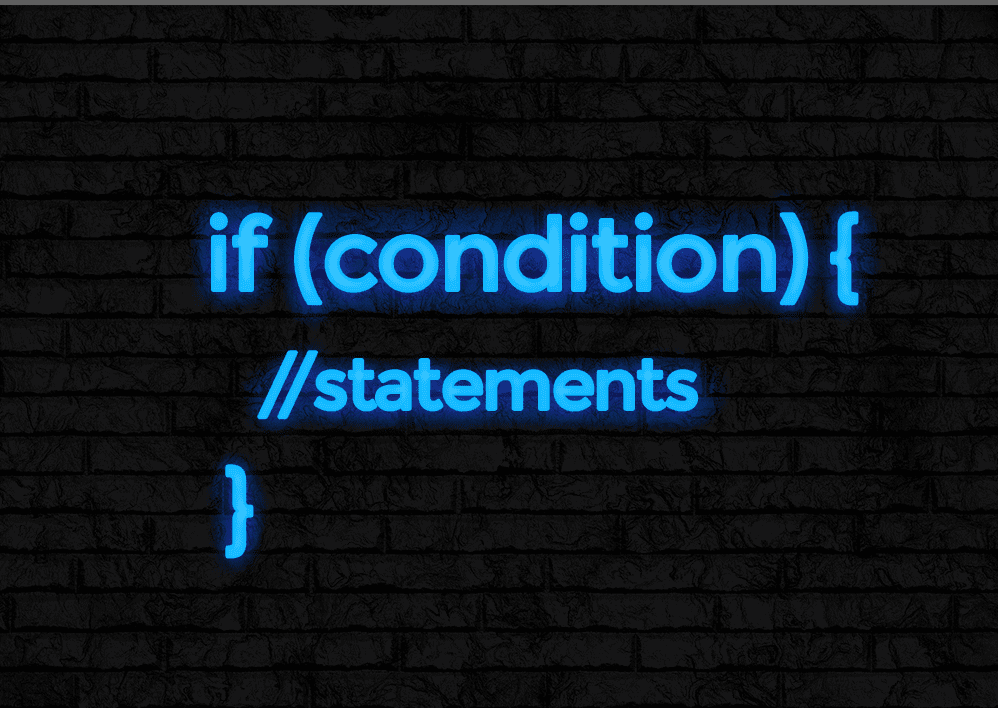
An if statement in Java is a one way conditional statement that decides the execution path based on whether the condition is true or false.
It’s a basic form of decision making in a program.
Join other Subscribers on our YouTube channel and enjoy daily pogramming tutorials.
Syntax of the if statement
1 2 3 |
if(booleanExpression) { //statements } |
A boolean is a data type in java. It has two data types. It’s either true or false.
Thus, a Boolean expression is an expression that resolves to either true or false.
If the boolean expression is true, the statements under the if statement are executed.
Relational Operators
Relational operators are usually used to form the boolean expression.
The relational operators include:
>
<
>= NOTE: > precedes =. No space between them
<= NOTE: == is equal sign. = is assignment operator
== equal to
! = not equal to
Recommended
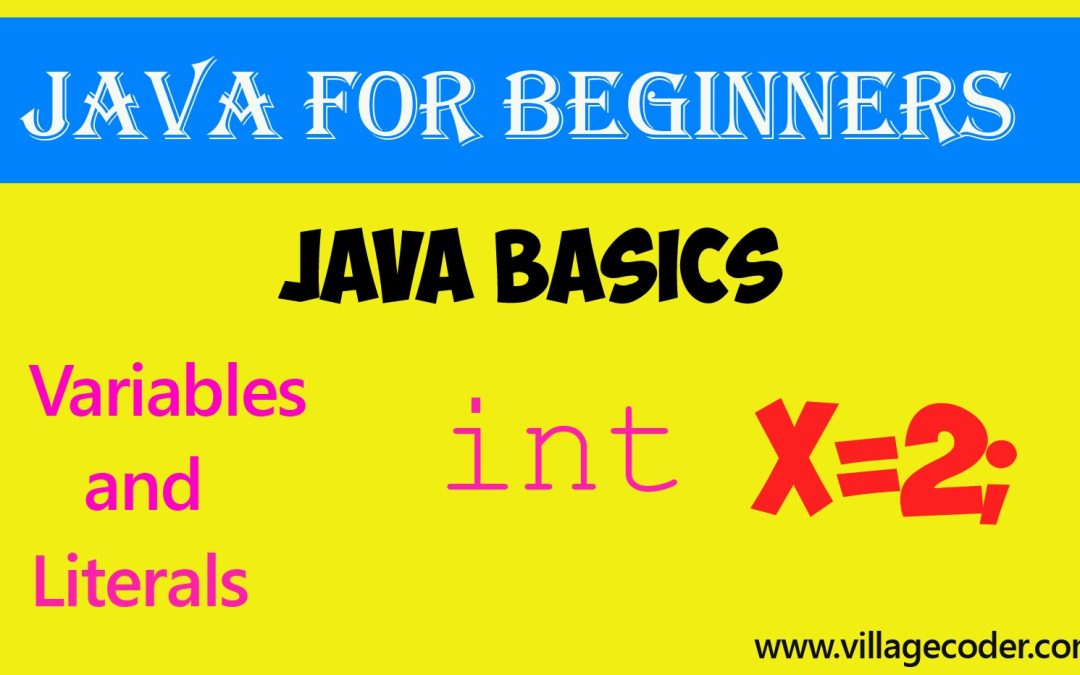
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
Examples of boolean expressions using relational operators
(1) The greater than operator.
Example : (10 > 5) is true
1 2 |
System.out.println(10 > 5); gives true System.out.println(10 > 55); gives false |
Let’s put it into an if statement.
1 2 |
if(10 > 5) System.out.println("10 is greater than 5"); |
(2) Less than (<).
Example : (4 < 9) is true
(42 < 9) is false
1 2 3 |
System.out.println(10 > 5); gives true System.out.println(10 > 55); gives false |
(3) Equal to (==)
In math, equal to sign is written as = but in Programming, particularly Java, we use double equal to signs. That’s ==. The = is called assignment sign in Java.
Example : x=5 means 5 is assigned to x or 5 is stored in x.
However, x==5 is read as x equals 5.
Example of boolean expressions using == include:
(3==3) resolves to true
(3==33) resolves to false
Let’s consider an if statement using the == relational operator :
1 2 |
if(4==4) System.out.println("It's a true statement"); |
(4) Not equal to (!=)
Examples of boolean expressions involving the != sign are:
(4 != 6) resolves to true
(7 != 7) resolves to false
(5) Greater than or equal to ( >=)
Examples of boolean expressions involving the (>=) sign are:
(9 >= 4) resolves to true
(9 >= 45) resolves to false
Let’s consider an if statement involving the ( >= ) sign :
1 2 |
if(9 >= 4) System.out.println("9 is truly greater than or equal to 4"); |
(6) Less than or equal to (<=)
Examples of boolean expressions involving the (<=)sign are:
(9 <= 4) resolves to false
(9 <= 45) resolves to true
Let’s consider an if statement involving the ( <= ) sign :
1 2 |
if(9 <= 45) System.out.println("9 is truly lesser than or equal to 45"); |
Program that demonstrates the use of variable in an if statement.
1 2 3 |
int value=10; if(value >5) System.out.println("value is greater than 5"); |
The boolean expression is sufficient
Many beginners wonder why the if statement is not something like this:
1 2 3 |
if(booleanExpression==true) { //statements } |
if (booleanExpression is true) or more appropriately,
if (booleanExpression == true)
But we just write something like this:
1 2 3 |
if(booleanExpression) { //statements } |
As a matter of fact, let’s state emphatically that the value of a boolean variable is sufficient, by itself, to control the if statement.
Example, consider this if condition :
1 2 |
if ( 40 > 10) System.out.println("40 is greater than 10"); |
If you consider the conditional statement, 40 > 10, it’s true that 40 is greater than 10. This means, 40 >10 resolves to true.
Thus, the above statement resolves to :
1 2 |
if ( true ) System.out.println("40 is greater than 10"); |
if we were to write :
1 |
if ((40 > 10 ) == true) |
then that would resolve to:
1 2 |
if( true == true ) System.out.println("40 is greater than 10"); |
This makes the statement,
1 |
if ((40 > 10 ) == true) |
superfluous or an over-kill.
Command flow of Java programs
All Java programs execute from head to bottom in a sequential manner. Example:
1 2 3 4 5 6 7 8 |
public class SequentialFlowProgram { public static void main(String[] args) { int salary=400; System.out.println ("You are a salary worker."); System.out.println("You qualify for a loan."); System.out.println ("We hope to see you again."); } } |
Output
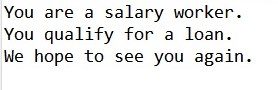
What if we want some block of code to execute only if a certain condition is present?
In that program above, all salary workers qualified for a loan. They could all take loans.
What if we decide in the future that only workers with a minimum amount of salary could take the loan? This can be done by using an *if statement*. Let’s rewrite the above program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
public class IfConditionProgram { public static void main(String[] args) { int salary=600; if(salary > 500){ System.out.println("You are IN the 'if' statement."); System.out.println("You are a salary worker."); System.out.println ("You qualify for a loan."); } System.out.println("You are OUT of the if statement."); System.out.println ("We hope to see you again."); } } |
Ouput
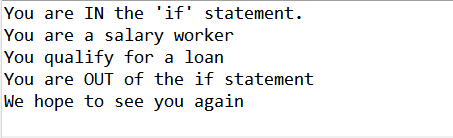
In the program above, the variable salary is assigned to the value 600 which greater than 500. Hence, the if statement is true
This means, statements in the if statement are executed.
What if the boolean expression of the if statement is false?
Let’s consider a condition where the if statment is false.
In the program below, the variable salary is assigned to the value 400 which NOT greater than 500. Hence, the if statement is false
This means, statements in the if statment are not executed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
public class IfConditionFalseProgram { public static void main(String[] args) { int salary=400; if(salary > 500){ System.out.println("You are IN the 'if' statement."); System.out.println("You are a salary worker."); System.out.println ("You qualify for a loan."); } System.out.println("You are OUT of the if statement."); System.out.println ("We hope to see you again."); } } |
Output
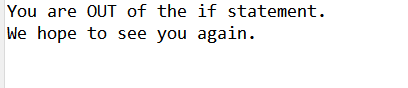
The if statement executes code only if its boolean expression is true
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public class TestingIfConditionProgram { public static void main(String[] args) { if(true) //The boolean expression is true so statement under it will execute and be displayed System.out.println ("This is a test expression"); if(false) //The boolean expression is false so statement under it will NOT. Nothing will be displayed. System.out.println("This is a test expression"); if(10 > 5) // 10 is greater than 5. This makes the boolean expression true so statement under it will execute and be displayed System.out.println("10 is greater than 5"); if(10 > 5) // 10 is NOT less than 5. This makes the boolean expression false so statement under it will NOT execute. Nothing will be displayed System.out.println("10 is greater than 5"); } } |
Variables can be used in the boolean expression
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
public class VariablesInIfConditionProgram { public static void main(String[] args) { int x=10; if(x > 5) //x holds the value of 10. Since 10>5, the expression x>5 evaluates to true. System.out.println("10 is greater than 5"); //Statement is executed to display "10 is greater than 5" /*Note that this if statement will NOT execute because y>5 evaluates to false */ int y=1; if(y > ;5) //y holds the value of 1. Since 1>5 is NOT true, the expression y > 5 evaluates to false. System.out.println("1 is greater than 5"); //Statement is NOT executed } } |
Ouput

When the if statement has more than one statement under it.
When the if statement has more than one statement under it, you have to introduce opening and closing braces around the statements.
When the statements of the if statement are not put in braces, only the first statement is considered connected to the if statement.
if statement with more than one statement must have curly braces
When the if statement does not have curly braces, only the first statement is executed.
The program below illustrates scenario where the boolean expression is true, the if statement has no curly brace.
1 2 3 4 5 6 7 8 9 10 11 12 |
public class ifStatementWithoutBraces { public static void main(String[] args) { if(true) System.out.println ("This statement executes under the if condition\n"); //this statement is connected to the if statement.It only executes //if the boolean expression is true System.out.println ("This statement DOES NOT execute under the if condition"); //This statement is not connected to the if statement. //It executes independent of the if condition or statement } } |
Ouput

This program below illustrates a scenario where the boolean expression is false, the if statement has no curly brace.
1 2 3 4 5 6 7 8 9 10 11 12 |
public class ifStatementWithoutBraces { public static void main(String[] args) { if(false) System.out.println ("This statement executes under the if condition\n"); //this statement is connected to the if statement.It DOES NOT //execute because boolean expression is false System.out.println ("This statement DOES NOT execute under the if condition"); //This statement is not connected to the if statement. //It executes independent of the if condition or statement } } |
Ouput

Don’t put semi-colon at end of boolean expression of ‘ if ‘ statement.
1 2 3 4 5 6 7 8 9 |
public class ifStatementWithoutBraces { public static void main(String[] args) { if(2 > 10); // Putting a semi-colon after the boolean expression makes it a null statement. //This disconnects the if condition from statements under it. System.out.println("2 is greater than 10"); //This statement executes independent of the if //statement. } } } |
Ouput

This statement shouldn’t have executed because (2>10) is false. However, because there’s semicolon at end of the if boolean condition, the if statement is disconnected from the if condition.
This implies the statement executed independently outside the scope of the if statement. That is, whether the boolean expression is true or false.
Using many ‘if ‘ statements in program to print a grade
You can use as many if statements as you want. The following program will look at how we can use many if statements to print grade of a student.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/*This program demonstrates the use of many if statements to display the grade of a * student based on his/her mark */ public class ManyIfStatements { public static void main(String[] args) { int mark =56; //mark of student char grade='F'; if(mark >20) grade='E'; if(mark >30) grade='D'; if(mark >40) grade='C'; if(mark >50) grade='B'; if(mark >60) grade='A'; System.out.println ("Grade is " + grade); } } |
Output
Grade is B
All if statements will be tested until the suitable if statement is reached and executed. This could be an inefficient way of printing the student grades.
Using ‘if’ statement in a ‘for’ loop
1 2 3 4 5 6 7 8 9 10 11 12 13 |
/*This program demonstrates the use of if statement in a for loop */ public class IfStatementInForLoop { public static void main(String[] args) { for(int i=0; i < 10; i++){ if((i % 2) == 0) // if the value of i is even, it is printed, else it is bypassed. System.out.println(i); } } } |
Output
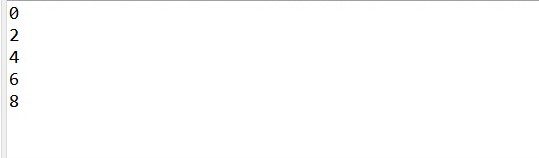
In the above code, the for loop iterates 10 times and at each iteration, the if statement checks whether the value of i is an even number. If it is an even number,the boolean expression evaluates to true and the statements under it are executed.
The statement (i % 2) == 0 means that the value of i is divided by 2 and its remainder is checked if it is equal to zero. If remainder is zero, then the number is an even number. Note that an even number is a number that 2 divides without a remainder.
Putting a semi-colon before the ‘if’ statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
/*This program demonstrates the use of if statement in a for loop. * NOTE: A semi-colon is put at the if statement. This makes the if statement null * and detaches the statement under it from the the if condition. H*/ public class IfStatementInForLoop { public static void main(String[] args) { for(int i=0; i < 10; i++){ if((i % 2) == 0); // semi-colon placed here makes if statement null. System.out.println(i); //All values of i are now printed because the if statement doesn't control //it anymore. } } } |
In the above program, we have placed a semi-colon at the end of the if statement. This makes the if statement is null detaching the statement under the if statement from it. Thus, we see that the output spans numbers 0, 1, 2, 3, 4, 5, 6, 7, 8, 9. This is because the if statement no more screens for only even numbers unlike the previous program.
Negating boolean expressions of ‘if ‘ Statements
If you negate the boolean expression of an if statement, the results turn from true to false or from false to true.
That’s;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
*This program demonstrates how the boolean expression changes from true to false and vice versa * if negated with the NOT operator*/ public class NegatingBooleanExpression { public static void main(String[] args) { if(true) System.out.println("This first statement will print"); //Now we negate the boolean expression- //This if statement will NOT execute because the boolean expression will be changed to false if(!true) System.out.println("This second statement won't print"); //Here, we negate the boolean expression, false, which turns to true- //This if statement will execute because the boolean expression will be changed to true if(!false) System.out.println("This third statement will print"); } } |
Output
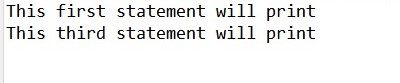
Let’s look at a typical Example:
1 2 3 4 5 6 7 8 9 10 11 12 |
/*This program has an if statement with its boolean expression not negated*/ public class BooleanExpressionNotNegated { public static void main(String[] args) { int age=35; if (age < 50) System.out.println ("Age is less than 50"); } } |
Now, let’s negate the boolean expression and see its results.
1 2 3 4 5 6 7 8 9 10 11 12 |
/*This program has an if statement with its boolean expression not negated*/ public class BooleanExpressionNegated { public static void main(String[] args) { int age=35; if !(age < 50) System.out.println ("Age is less than 50"); } } |
You May Also Like…
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point number of 7 digits of...
Recent Comments