Basic Parts of a Java program
In Java, whenever you create an object from a class, the object is called an instance of the class. The object is something like a child of the class and it holds characteristics of the class just as a child will have characteristics of the parent. Each object is different and has its own copy of the methods the class (parent) has.
nanadwumor
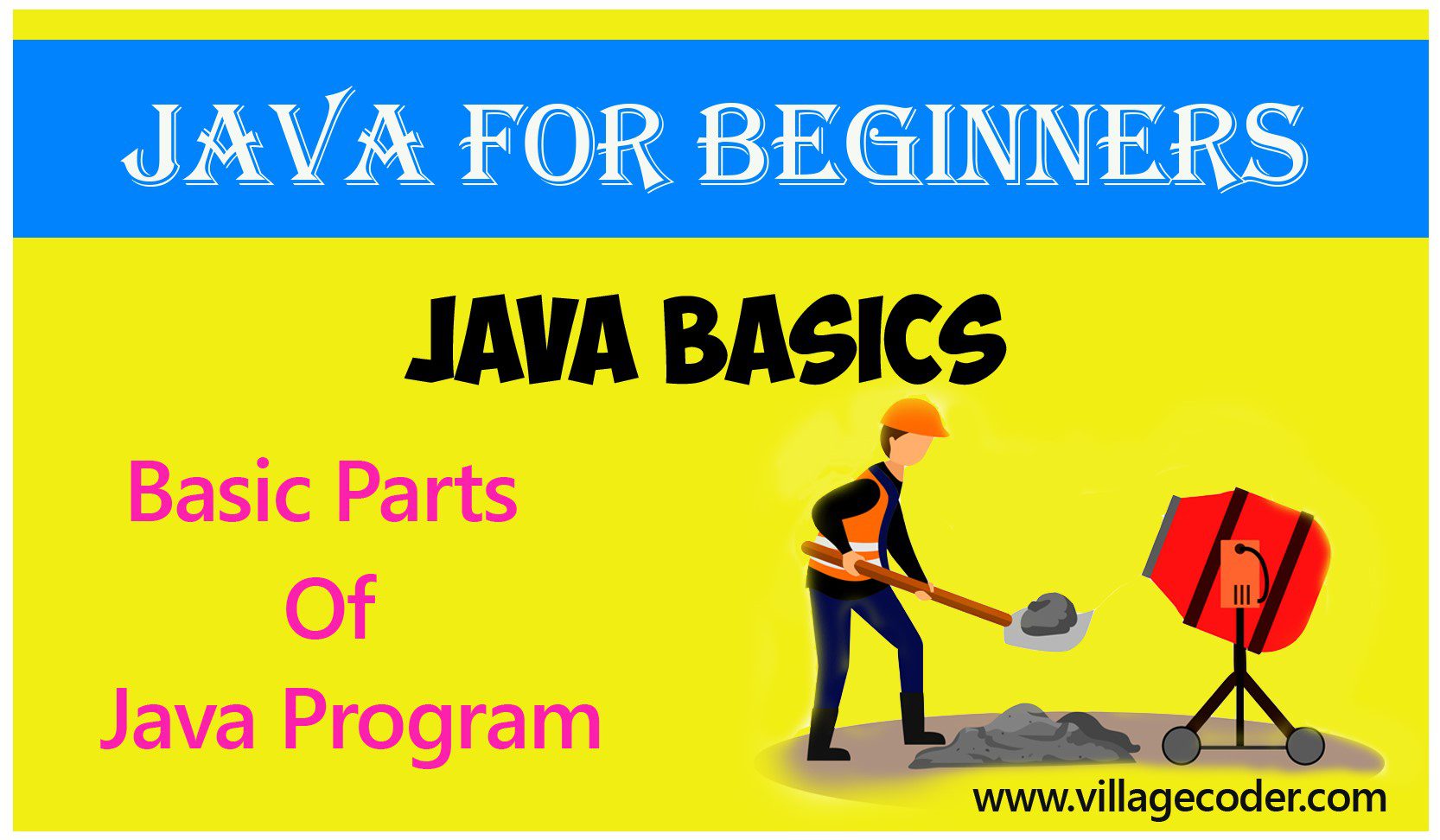
- Java language consists of specific parts such as class, methods, variables etc
- The main method is the point of start of a Java program
- Java has keywords that are reserved for special function
RECOMMENDED ARTICLES
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures. In Java, a variable is a container used to store...
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal number. The double data type is a floating point number. It is double precision data type The range is 4.9406564584124654 x...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These methods are part of the Java API. The print and println methods are used to print string of characters onto the console The print...
A Java program consists of parts that have specified functions. For Instance, Java program has a class name, access specifiers, variables, methods etc
Let’s look at what a basic Java program looks like and its parts.
Join Other Subscribers on Our YouTube Channel and Don’t Miss a thing!
Basic Java Program
1 2 3 4 5 6 7 8 9 10 |
//This is a basic Java program public class BasicProgram { public static void main(String[] args) { //opening brace for main method int x=2; System.out.println("The square of " + x + " is " + x*x); }//closing brace for main method } |
Output
The square of 2 is 4
Explanation
The program above is stored as BasicProgram.java. Java programs are stored as a .java file and the file name must be the name of the class.
The class name is BasicProgram so the file is stored as BasicProgram.java.
All Java programs must be stored with a .java extension. This helps the compiler to identify Java code and execute. Once again, this is why our program is stored as BasicProgram.java
Let’s look at the individual lines of code.
Line 1:
//This is a basic Java program
The two forward slashes // are used to add a comment to your Java code. Comments are ordinary human statements the Java programmer adds to his code to explain the meaning or function of the line of code.
In the above example, the Java compiler will note the // and treat the succeeding statement “This is a basic Java Program” as not part of the code but just something to add some explanation to the code in order to help others understand the code, or even the programmer understand the code in the future if not in the present.
Comments are optional so the programmer may never comment his lines of code. However, it is very advisable to add comments to your code in order to help explain what your statements are executing.
Why should you comment your code?
Programs in real life are long and complex than this basic example so adding comments will help you understand your own code.
Imagine writing 3,000 lines of code without comments and after five years, you pick the code to make changes or improve it. Will you remember everything? Certainly not. Isn’t it? So, always comment your code as a good practice.
Line 2:
Line 2 is an empty space. There are no characters on this line. Just as we write sentences and leave blank spaces for our statements to be legible and easily readable, blank spaces are inserted in code by programmers in order to make their code easily readable.
In other words, the blank space has no other function other than making the code look neat and easily readable. It’s a good practice to write human-readable code.
Line 3:
public class BasicProgram
At line 3, the actual program begins. This line contains the class header. The class header consists of the class name, the keyword, class and the access specifier, public. The keyword class tells us that BasicProgram is the name of the class.
A Java program comprises classes. A class is used to create an object. Java is an object-oriented programming language. This means, objects are used to execute functions in Java. And these objects are created from classes. Therefore, every Java program needs to have at least one class.
Let’s take a closer look at our class header.
public class BasicProgram
public : The first word we see is public. public is a Java keyword. A Java keyword is a word that has a special function in Java and thus, cannot be used by the programmer for any other purpose, such as being used as identifiers, unless what it’s programmed to be used for.
public is an access specifier. That’s, it specifies what program or code can get access to our class. If a class is public, then it means any code whether inside or outside the class package can access the class.
A public class is literally accessible to the public (pun intended).
There are other access specifiers such as public, protected, default.
Let’s look at some examples :
public class BasicProgram
This class has a public access specifier. This means our class can be accessed by any code.
private class BasicProgram
This class has a private access specifier. This means class is off limits to code outside
protected class BasicProgram
This class has a protected access specifier. This means class is accessible to code in the same package and subclasses to the BasicProgram class. In Java, a class can extend another class in a process called Inheritance.
class BasicProgram
This class has no access specifier. This means the class has a default or package access specifier. That’s, only code in the same package as our class can access BasicProgram.
TAKE NOTE: A Java file can have many classes but only one of the classes can be declared as public.
All keywords are reserved words for Java. All keywords or reserved words are typed in lowercase.
Java is a case-sensitive language. This means characters in lowercase are not the same as those in uppercase.
This implies that Public or PUBLIC will create an error. All letters must be in lowercase.
class : class is also a Java keyword and it’s used to show a class. Every class in Java must have the keyword class. The class keyword marks the beginning of a class definition.
BasicProgram : This is the class name. This name is what the programmer chooses to call his class. Although any name can be given to a class, most programmers name their classes such that the classes can easily be identified. For Instance, a class that calculates average marks of students may be called AverageMark.
Note that it’s not necessary to give names to a class based on what it does. It only helps the programmer to easily identify the purpose of his class among the many classes.
A class name is not a keyword or reserved word. It’s a programmer-defined word. The programmer cannot use any of Java’s keywords or reserved words as name of his class. This will cause an error.
A class name can be all lowercase or uppercase or mixture of both. A good practice though is to begin your class names with an uppercase.
Class names cannot begin with a number. Example : 3Party, 1touch are all unacceptable class names because they begin with numbers. This will cause the compiler to complain and an error will occur.
Names that the programmer gives to his classes are called identifiers. That’s, the names help to identify the different types of classes or group of code.
Line 4:
An opening brace follows immediately after a class name. This is found at line 4 in our program. The opening brace delimits the beginning of the body of the class. The closing brace at line 10 indicates the end of the body of the class.
CAUTION: If you fail to add corresponding closing brace to an opening brace, your program won’t compile and an error will occur. Always, remember to close your curly or opening braces.
Let’s take a second look at our program to note the opening and closing braces.
1 2 3 4 5 6 7 8 9 10 |
//This is a basic Java program public class BasicProgram { //class opening brace public static void main(String[] args) { int x=2; System.out.println("The square of " + x + " is " + x*x); } //class closing brace } |
Line 5:
public static void main(String[ ] args)
The line 5 contains a method header. Remember that we have said that Java consists of classes. A Java class may have methods which perform specific functions.
A method is a group of Java statements that have a specified function. For example, we can write a group of statements that square a number and give a name to it. This group of code is called a method.
Describing a method
A method contains some keywords that describe or qualify the method. Now, let’s look at our method header again.
public static void main(String[] args)
public : Just as a class can be public, a method too can also be public. public is an access specifier and it implies that the method can be accessed by any outside code.
That’s, the method is publicly accessible.
static : The word static means “not changing“. In Java, when we say a method is static, it means the method belongs to the class and not an instance of the class.
Let’s try to explain further.
In Java, whenever you create an object from a class, the object is called an instance of the class. The object is something like a child of the class and it holds characteristics of the class just as a child will have characteristics of the parent. Each object is different and has its own copy of the methods the class (parent) has.
However, there are instances where we want the method to belong to the class (parent) only such that an object (child) cannot have its own customized copy but all objects (children) will have to access this one (static) method belonging to the class (parent).
In such case, we make the method static implying the method is one for all objects and cannot be different for different objects. It’s static in its literal sense.
Line 6:
Line 6 has an opening brace again. This opening brace encloses the body code of the main method. Remember that each opening brace has a closing brace to enclose the code of the method’s body.
Let’s look at the main method again to note where its opening and closing braces are.
public static void main(String[ ] args)
{ //opening brace for main method
int x=2;
System.out.println(“The square of ” + x + ” is “ + x*x);
} //closing brace for main method
Line 7:
int x=2;
This line has the variable x declared as an int and initialized to 2. The int is a data type in Java. x is the name of the variable. This implies that x can reference only int values. int is short for Integer. It is a primitive data type.
Line 8:
System.out.prinln(“The square of “ + x ” is “ + x*x);
This statement displays The square of 2 is 4.
System is a class. out is an object serving as a field in the System class. println is a method of the out object.
Note: We pause here to state that this line of the code is the only line that prints something to the screen.
The message displayed on the screen is put in double quotation brackets. Statement put in double quotation brackets are called a String or String literal.
At the end of the statement is a semi-colon. A semi-colon ends a statement in Java just as a period is used to end human languages like English.
However, semi-colons are not used to end every statement in Java. Let’s look at statements in Java that do not warrant a semi-colon to end it.
(1) Comments : A comment does not need a semi-colon. A comment in Java is not part of the Java code. It only explains what the code does. Thus, it’ll be unnecessary to terminate a comment with a semi-colon as that semi-colon will be treated as part of the comment and ignored by the Java compiler.
(2) Class header : The class header does not end with a semi-colon. A class header begins a body of code so if it ends with a semi-colon, the header will be cut off from the body of code.
(3) Method header : Just like a class header, method headers don’t end with semi-colon. A method header begins a method followed by opening brace and ends with a closing brace. The method code is put within the braces. So a method is delimited by opening and closing curly braces.
(4) Curly braces: We don’t bring semi-colon after an opening or closing curly bracket. Curly braces are used to contain a group of code in a class or method. The opening brace signifies the beginning of the code and the closing brace shows the end of the code.
Characters used in the program
There are a couple of characters that were used in our BasicProgram class. Let’s take a look at them and their use.
(1) // : Double slash – The double slash indicates the beginning of a comment
(2) { } : Opening and closing braces – Used to enclose a body of code. The body code of both a class and a method are enclosed in opening and closing braces.
(3) ” “ : Quotation marks – These are used to enclose a group of characters or a string literal. Characters in double quotation marks are called Strings or string literal.
(4) ( ): Opening and closing brackets – They are used in a method header. Methods that accept arguments have parameters in the brackets. These parameters accept the arguments.
(5) + : plus sign – The plus sign is used to concatenate or join two strings in Java. If the + is used to concatenate strings, the operand on the right is automatically converted into a character string before concatenated with operand on left.
Just like in Arithmetic, the plus sign is used to perform addition Operation. Example : 2+5 will give 7 in Java.
(6) ; Semi-colon – It’s used to indicate the end of a Java statement. Note that not all Java statements need a semi-colon.
Important tit-bits about a Java program
The following are important tit-bits about a Java program.
(1) A Java program is stored in a file with a .java extension. Example, our program was stored as BasicProgram.java
(2) A Java file can have many classes but only one class can be declared public. The file name must have the same name as the public class.
(3) Comments are not part of the Java code. They add explanation to the code. Hence, they are ignored by the Java compiler. Comments are preceded by double forward slash. Example :
//This is just a comment.
If you want to add several lines or comment, there is another way to do that. To add multi-comments, we first type a forward slash and asterisk.
We then type any number of lines of comments then type asterisk band forward slash to close it. Example:
/*
These lines are comments in Java.
They are ignored by the Java compiler.
Multi-comments are delimited by a forward slash and asterisk
*/
(4) Java is case-sensitive. That’s, lowercase letters are different from uppercase letters. Example, public is not the same Public.
(5) If you type an opening brace, you should add a closing brace to match it. This means, if you have 3 opening braces, you should also have 3 closing braces. That’s, for each opening brace, there must be a corresponding closing brace.
You May Also Like…
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
double data type in Java
The double data type is also a floating point number. It is called double because it has a...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the...
0 Comments