An exception is a problem that arises during the execution of a program. Exception is a fancy word for a disruption or error in program execution.
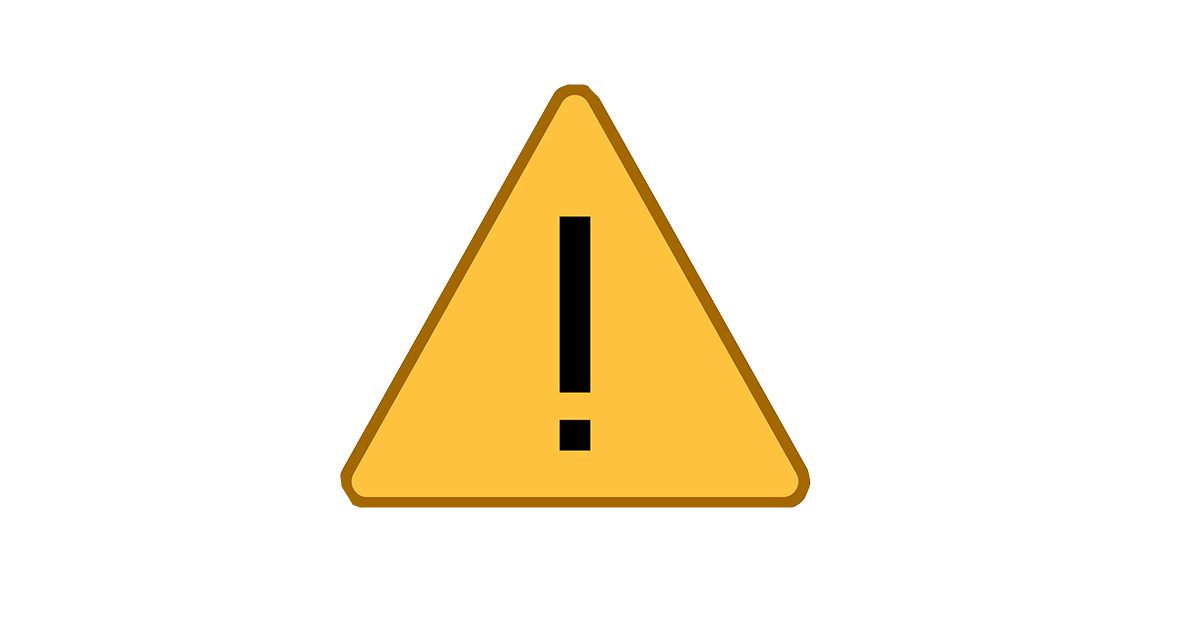
An exception is a problem that arises during the execution of a java program.
Exception is a fancy word for a disruption or error in program execution.
When an Exception occurs, the normal flow of a program becomes disrupted and the program terminates abnormally.
Join other Subscribers on our YouTube channel and enjoy daily pogramming tutorials.
Program demonstrates the occurrence of an exception
Let’s look at an example of an exception that occurs during the execution of a program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
/* * Program demonstrates the occurence of exception at index 3 because it doesn't exist. */ public class ExceptionDemo { public static void main(String[] args) { //create an array with 3 elements int[] numbers= {55,78,36}; //attempt to read beyond the bounds of the array for(int i=0; i<=3; i++) { System.out.println(numbers[i]); } } } |
Recommended
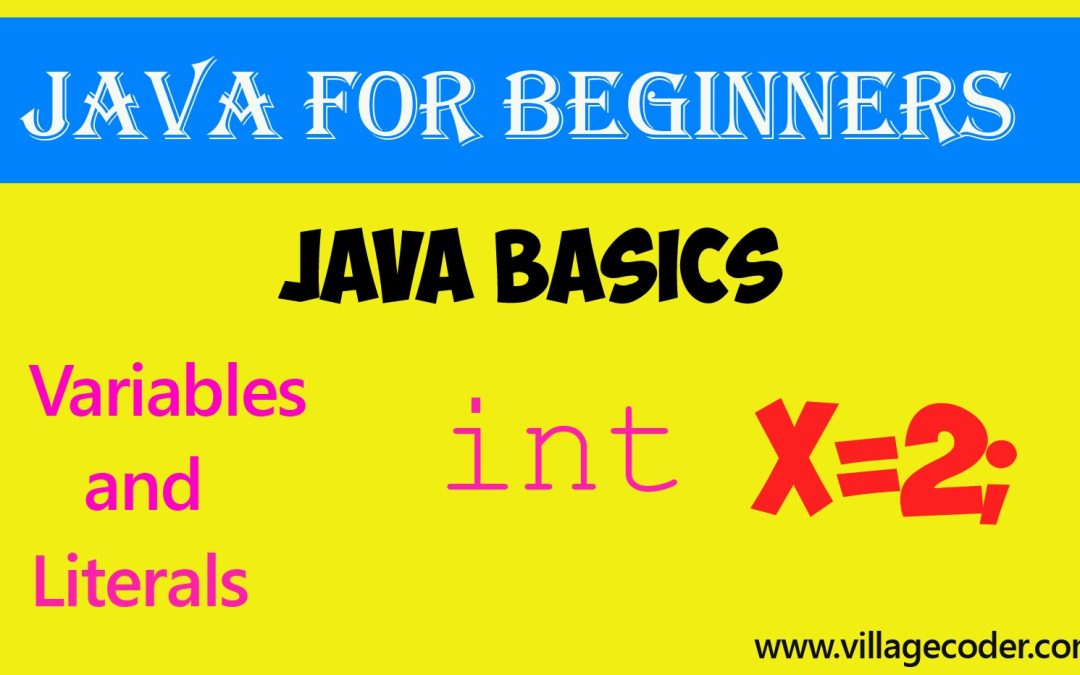
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
Output

Explanation of program
The program above crashes because the array has the indexes 0, 1, 2 only and there is no index at number 3.
The array, numbers, has the elements 55, 78, 36 which have indices at 0,1 and 2 respectively.
That is, the element 55 is at index 0.
The element 78 is at index 1.
The element 36 is at index 2.
However, the for loop reads from 0, 1, 2 up to 3. The index 3 is non-existent so when the program attempts to access index 3, it crashes.
Therefore, the program crashes when it tries to access or read the element at numbers[3]. It displays an error message which is similar to the one above. This is called Exception.
Exception Handler
When an error occurs within a method, the method creates an object. The type of object is an Exception or Exceptional object.
The exceptional event or object (exception) is handed off to the runtime system. The object contains information about the error.
This information contains its type and the state of the program when the error occurred.
Throwing an Exception
The process of creating an exception object and handing it to the runtime system is called throwing an exception.
What happens if an exception occurs?
During the execution of a program, when a method encounters a problem, it throws an exception.
When this happens, the Java runtime system attempts to find something to handle the exception.
The call stack
In a typical java program, one method calls the other in succession. Normally, it begins with the main method calling another method which intend calls a second method, and third, and fourth in that order.
This list of methods is what we call the Call Stack.
Let’s look at a program that prints the call stack or the stack trace.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/** * This program demonstrates the stack trace that is produced when an exception occurs * when a program is executing. */ public class StackTrace { public static void main(String[] args) { System.out.println("In the main method now."); System.out.println("calling firstMethod..."); firstMethod(); System.out.println("main Method has finished executing."); } /** * firstMethod */ public static void firstMethod() { System.out.println("In the firstMethod now."); System.out.println("Calling createError method..."); createError(); System.out.println("myMethod has finished executing."); } /** * createError */ public static void createError() { String str="abc"; System.out.println("In createError method now."); //this statement causes an exception to occur System.out.println(str.charAt(3)); System.out.println("createError has finished executing."); } } |
Output
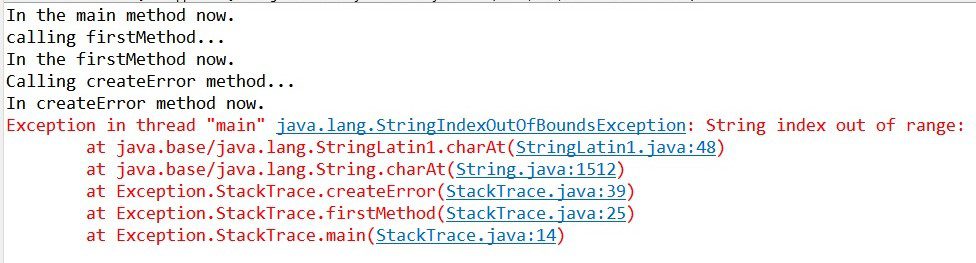
How Does JVM handle an Exception?
When an exception occurs in a method while the method is executing, the method halts and creates an exceptional object. This object is thrown to the runtime system (JVM).
The exception object contains the name, description, current state and place of occurrence of the exception in the program.
The Java runtime system searches the call stack to fish out the method that contains a block of code that can handle the exception that has occurred. This block of code is called an exception handler.
After occurrence of the exception, the Java runtime quickly takes note of the method in which the exception or problem occurred.
From here, it finds out if that method which caused the exception has an exception handler, that’s any block of code capable of dealing with the exception.
If this method lacks any suitable exception handler, the JVM throws the exception back to the preceding method that called the current method.
Again, the JVM checks if this preceding method has any exception handlers for the exception or disruption. If it has, the issue is handled gracefully by the exception handler.
However, if it doesn’t have, the exception or problem is thrown back again to another preceding method. If the said method happens to have a suitable exception handler, it tackles the problem and resolves it.
However, if not, the throw-back continues through the preceding methods in the reverse order.
Note that a suitable exception handler means the type of handler (or block of code) matches the type of the exception object created or thrown.
The run-time system starts searching from the method in which the exception occurred, and proceeds through the call stack in the reverse order in which methods were called.
If it finds an appropriate handler then it passes the occurred exception to it. An appropriate handler means the type of the exception object thrown matches the type of the exception object it can handle.
Program demonstrates how JVM throws Exception through the methods.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
public class HandlingException { public static void main(String[] args) { System.out.println("In the main method now."); System.out.println("calling firstMethod..."); try { firstMethod(); System.out.println("main Method has finished executing."); } catch (Exception e) { System.out.println("The Exception occured in createError method but it is handled " + "here in the main method"); } } /** * firstMethod */ public static void firstMethod() { System.out.println("In the firstMethod now."); System.out.println("Calling createError method..."); createError(); System.out.println("myMethod has finished executing."); } /** * createError */ public static void createError() { String str="abc"; System.out.println("In createError method now."); //this statement causes an exception to occur System.out.println(str.charAt(3)); System.out.println("createError has finished executing."); } } |
Explanation of code
The program execution begins in the main method. The statements in the main method are executed.
The try statement is executed. Within the try statement, the firstMethod() executes. Program jumps to the firstMethod().
1 2 3 4 5 6 7 8 9 |
/** * firstMethod */ public static void firstMethod() { System.out.println("In the firstMethod now."); System.out.println("Calling createError method..."); createError(); System.out.println("myMethod has finished executing."); } |
Statements in the firstMethod() are executed. The createError() method executes. This skips the execution to the createError() method.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
/** * createError */ public static void createError() { String str="abc"; System.out.println("In createError method now."); //this statement causes an exception to occur System.out.println(str.charAt(3)); System.out.println("createError has finished executing."); } |
Statements in the createError() method are executed. An error occurs in the statement
System.out.println(str.charAt(3));
1 2 |
//this statement causes an exception to occur System.out.println(str.charAt(3)); |
This is because the str string has only 3 characters and there is no character at the index 3. Note that character ate index 3 is the fourth character as index numbering starts from 0.
The charAt() method creates an Exception. The JVM looks for an Exception handler withing the createError() method. This exception has no Exception handler to handle the error.
The JVM thus throws the exception to the method that called the createError() method. This is the firstMethod(). The JVM does not find any exception handler in the firstMethod() to handler the error or exception.
If further throws it to the method that called the firstMethod(). This is the main method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
public static void main(String[] args) { System.out.println("In the main method now."); System.out.println("calling firstMethod..."); try { firstMethod(); System.out.println("main Method has finished executing."); } catch (Exception e) { System.out.println("The Exception occured in createError method but it is handled " + "here in the main method"); } } |
Fortunately, there is a try-catch expression in the main method and it can handle our exception.
Note that the catch expression accepts Exception as argument. That is, it accepts all exceptions.
This Exception handler catches the exception gracefully and prints out the message:
The Exception occured in createError method but it is handled here in the main method
Output

If the JVM fails to find a suitable exception handler for the exception thrown
After searching through all the methods on the Call Stack, if the run-time system fails to find any suitable handler, the Java run-time system hands over the Exception Object to the default exception handler.
The default Exception handler is already part of the Java runtime system. The default Exception handler will halt the program and prints out a message which describes the type or nature of the exception. This terminates the program abnormally.
Program demonstrates how an exception is handled by the default Exception handler
Let’s look at a scenario that the runtime system finds a suitable exception handler to handle the exception and gracefully end the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
/** * Program demonstrates occurrence of ArithmeticException. Program crashes and JVM * gives a default message about the error. * */ public class ExceptionDemo { public static void main(String[] args) { int x = 12, y = 0; int z = x/y; // cannot divide by zero System.out.println ("Result = " + z); System.out.println ("Sorry. You cannot divide a number by 0"); } } |
Output

ArithmeticException is thrown as the program attempts to divide by zero. Since there is no Exception handler, the JVM handles it and thorws the above message noting that there is Exception in the main method or thread. It names the type of Exception as ArithmeticException.
Writing your own exception handler to handle exceptions
It’s advisable to write Exception handlers to handle potential exceptions in your program.
If the programmer fails, his program will crash and exit abruptly causing bad user experience.
To handle exceptions, Java programmers use keywords such as try, catch, throw, throws and finally. Let’s understand each keyword:
try: The block of code that is likely or suspected to throw an exception is placed in the try block. As the name suggests, the JVM try to run this program to see if there will be any exceptions or errors.
catch: As the name suggests, the catch phrase catches the exceptions that are thrown by then try statement. It catches the exceptions and gracefully handles them without causing the program to halt abruptly.
Exceptions generated in the course of program execution are thrown by the Java run-time system automatically.
throw: Java has a host of exceptions it automatically recognizes and throw it if encountered.
Example is the ArrayIndexOutOfBoundsException. Java runtime system will complain if you try to read elements in the array beyond the length.
However, Java cannot possibly think of all the exceptions in the world. The programmer who develops computer games may want his character to take certain defined trajectory, if a player of the game does otherwise, that is against the programmer-defined rules.
In an instance like this, the programmer can create a new Exception by extending Java’s default Exception class. Because this type of disruption is not recognized by the JVM by default, the programmer has to manually “throw” the exception.
The throw keyword is used to throw exceptions manually even if the JVM hasn’t complained. This could be one of Java’s predefined exceptions or programmer-defined exception. It’s used within the method’s block of code.
throws: The throws keyword is different from the *throw* keyword explained above.
At a glance or on the surface, the play of words is evident – one is a singular verb (throws) and the other is a plural verb(throw).
The throws keyword is used in the method’s signature to show that the method has some block of code capable of throwing exceptions.
Read More : Difference between the throw and throws keyword
finally: In what we call a try-catch statement, the finally keyword ensures that a block of code is executed even after completion of the try or catch statement.
Handling exceptions with try-catch statement
The program below handles an ArrayIndexOutOfBoundsException with a try-catch statement gracefully.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/* * Program demonstrates the use of a try-catch statement to arrest * the occurence of exception at index 3 because it doesn't exist. */ public class Exception { public static void main(String[] args) { //create an array with 3 elements int[] numbers= {1,2,3}; try { //attempt to read beyond the bounds of the array for(int i=0; i<=3; i++) { System.out.println(numbers[i]); } } catch(ArrayIndexOutOfBoundsException e){ System.out.println(e.getMessage()); } } } |
Output
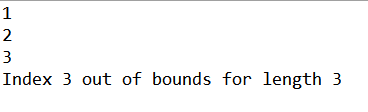
Explanation of code
An array of integers is declared. This is called numbers. The array contains the elements 1,2,3. Thsese elements are at the indexes 0, 1 and 2.
The try statement executes. The for loop executes and prints out the elements in the array at every iteration. It iterates at values of i=0,1,2,3.
However, the numbers array has length of 3 and hence, does not have any element at index 3.
An attempt to access index 3 throws an ArrayIndexOutOutBoundsException. This exception is caught by a corresponding catch statement and the statement in the catch expression executed.
e.getMessage() prints the default message for the ArithmeticException.
Important points to remember about Exception
(1) In a single method, there may be more than one statement that throws different types of exceptions.
Put each block of statement that will throw a specific exception in its own try block and provide a catch statement for each.
(2) A try block should be followed by a catch block. The catch block is the exception handler.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
public class ArithmeticExceptionDemo { public static void main(String[] args) { try { int x = 12, y = 0; int z = x/y; // cannot divide by zero System.out.println ("Result = " + z); } catch(ArithmeticException e) { System.out.println ("Sorry. You cannot divide a number by 0"); } } } |
(3) There can be zero or more than one catch block for one try block. This is possible because within one try block, there could be more than one exception thrown.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/** * Program demonstrates multicatch statements for a try statement. * */ public class MultiDemo { public static void main(String[] args) { try { int[] arr=new int[4]; arr[4]=12; //attempt to put 12 into index 4 creates ArrayIndexOutOfBoundsException int x=8/0; //attempt to divide by 0 creates ArithmeticException } catch (ArithmeticException e) { System.out.println("Artithmetic Exception: You cannot divide by 0"); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Array Index Out Of Bounds Exception: index 4 in INVALID."); } catch (Exception e) { System.out.println("Main Exception: This is the Superclass Exception."); } } } |
(4) One try-catch block can be nested in the other.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/** * Program demonstrates one try-catch statement nested in the other * */ public class NestedTryCatch { public static void main(String[] args) { try { int x=8/0; //attempt to divide by 0 creates ArithmeticException } catch (ArithmeticException e1) { System.out.println("Artithmetic Exception: You cannot divide by 0"); System.out.println(); //print a space try { int[] arr=new int[4]; arr[4]=12; //attempt to put 12 into index 4 creates ArrayIndexOutOfBoundsException } catch(ArrayIndexOutOfBoundsException e2) { System.out.println("Array Index Out Of Bounds Exception: 4 is an INVALID array index"); } } } } |
(4) All exceptions inherit from the Exception class which in turn inherits from the Throw able class.
(5) The finally keyword is optional. The finally statement always get executed whether an exception occurred in the try block or not. If an exception occurs in the try block, the catch block executes before the finally block executes.
However, if there are no exceptions in the try block, the finally block executes after execution of code in the try block.
The finally block is used to put important codes such as clean up code.
You May Also Like…
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point number of 7 digits of...
0 Comments