How to add or subtract two matrices
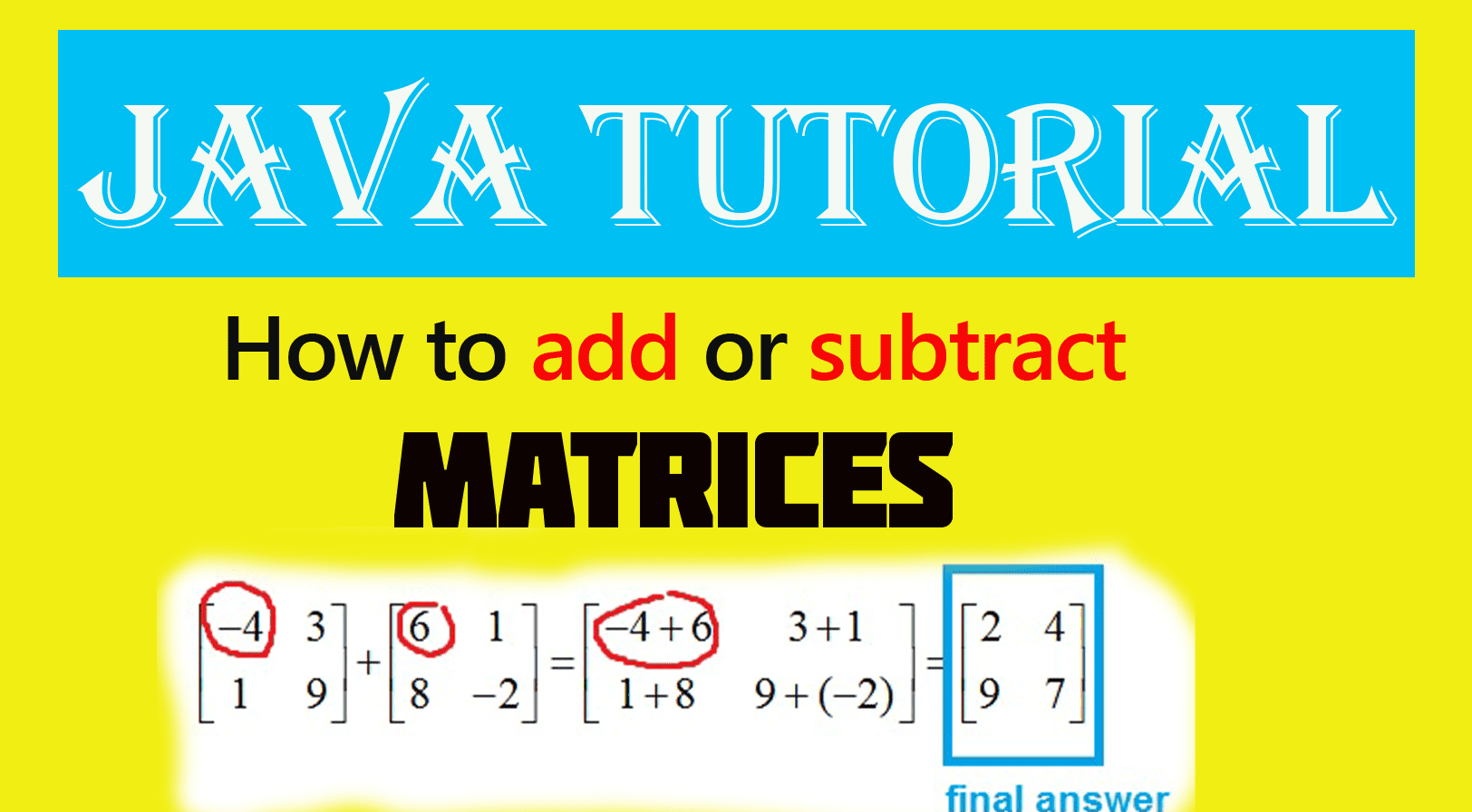
This program illustrates how to add or subtract two compatible matrices of any size. The idea is very simple. We will create three 2-dimensional arrays. The first two arrays will hold the elements of the two matrices. The third 2-dimensional array will hold the elements of the sum or difference of the two matrices.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
import java.util.Scanner; //needed for the Scanner class /* * This program adds or subtracts two compatible matrices */ public class Matrices { public static void main(String[] args){ //declare two variables to take inputs for the row and column of the matrix int rows, cols; //Create Scanner object to take inputs from keyboard Scanner in = new Scanner(System.in); System.out.print("Enter the number of rows: "); rows=in.nextInt(); System.out.print("Enter the number of columns: "); cols=in.next(); //leave a space System.out.println(); //Create three arrays to hold three matrices //First two arrrays hold the two matrices while third array holds the resulting matrix int[][] matOne=new int[rows][cols]; int[][] matTwo=new int[rows][cols]; //input the elements of the matrices System.out.println("Input the elements of the first Matrix"); for(int i=0; i<rows; i++){ for(int j=0; j<cols; J++){ System.out.print("row" + (1+i) + ",column" + (1+j) + ":" ); matOne[i][j]=in.nextInt(); } } //leave a space System.out.println(); //Input the elements of second matrix: MatTwo for(int i=0; i<rows; i++){ for(int j=0; j<cols; J++){ System.out.print("row" + (1+i) + ",column" + (1+j) + ":" ); matTwo[i][j]=in.nextInt(); } } //leave a space System.out.println(); //Add the members of the two matrices for(int i=0; i<rows; i++){ for(int j=0; j<cols; j++){ matSum[i][j]=matOne[i][j] + matTwo[i][j]; } } //Print the resulting matrix System.out.println("The resulting matrix is:"); for(int i=0; i<rows; i++){ for(int j=0; j<cols; j++){ System.out.print(matSum[i][j] + " "); } System.out.println(); //go to the next line } } } |
Subtracting two matrices
Subtracting one matrix from another matrix is pretty much similar to adding the two matrices except the change of the operation sign from plus to minus. The program below subtracts one matrix, MatTwo, from the other matrix, MatOne.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
import java.util.Scanner; //needed for the Scanner class /* * This program adds or subtracts two compatible matrices */ public class Matrices { public static void main(String[] args){ //declare two variables to take inputs for the row and column of the matrix int rows, cols; //Create Scanner object to take inputs from keyboard Scanner in = new Scanner(System.in); System.out.print("Enter the number of rows: "); rows=in.nextInt(); System.out.print("Enter the number of columns: "); cols=in.next(); //leave a space System.out.println(); //Create three arrays to hold three matrices //First two arrrays hold the two matrices while third array, MatDiff, holds the resulting matrix int[][] matOne=new int[rows][cols]; int[][] matTwo=new int[rows][cols]; int[][] matDiff=new int[rows][cols]; //input the elements of the matrices System.out.println("Input the elements of the first Matrix"); for(int i=0; i<rows; i++){ for(int j=0; j<cols; J++){ System.out.print("row" + (1+i) + ",column" + (1+j) + ":" ); matOne[i][j]=in.nextInt(); } } //leave a space System.out.println(); //Input the elements of second matrix: MatTwo for(int i=0; i<rows; i++){ for(int j=0; j<cols; J++){ System.out.print("row" + (1+i) + ",column" + (1+j) + ":" ); matTwo[i][j]=in.nextInt(); } } //leave a space System.out.println(); //subtract the members of the two matrices for(int i=0; i<rows; i++){ for(int j=0; j<cols; j++){ matDiff[i][j]=matOne[i][j] - matTwo[i][j]; } } //Print the resulting matrix System.out.println("The resulting matrix is:"); for(int i=0; i<rows; i++){ for(int j=0; j<cols; j++){ System.out.print(matSum[i][j] + " "); } System.out.println(); //go to the next line } } } |
Recent Comments