How to change the color of the background of a JFrame content pane
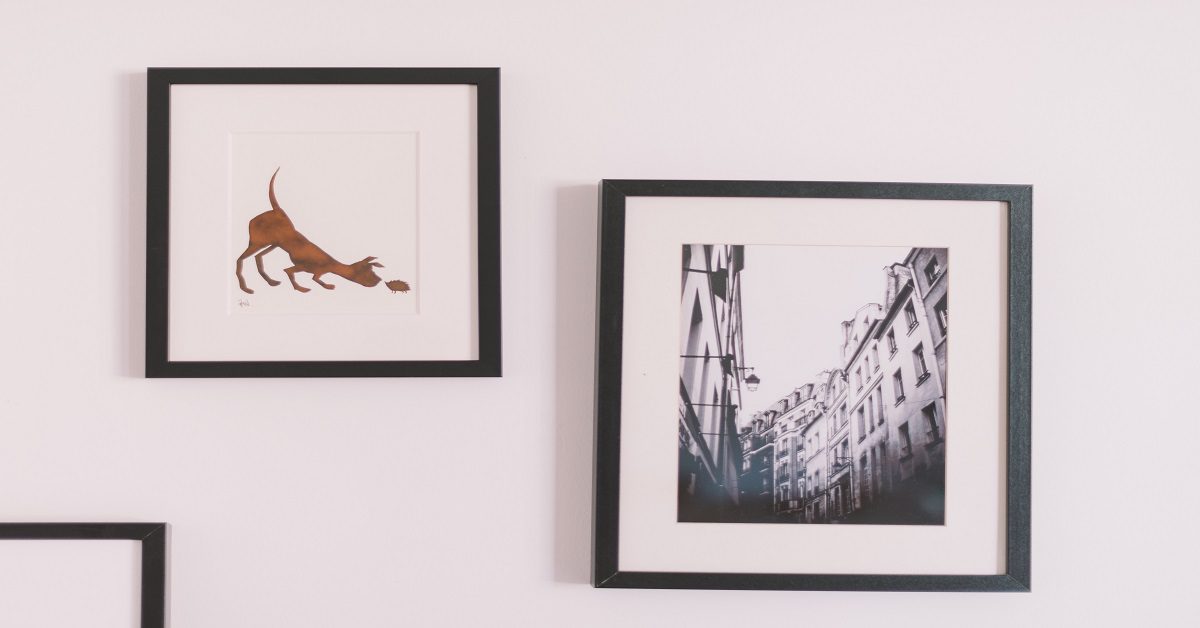
The JFrame has a content pane. When you add an object to a JFrame object, you are actually adding it to the content pane of the JFrame.
The content pane is a container the is part of every JFrame object. You cannot see content pane. It does not have a border but any component that is to be displayed in a JFrame must be added to its content pane.
A panel is also a container that can hold GUI components. Panels cannot be displayed by themselves unlike JFrame objects which can be displayed by themselves.
Panels are used to hold and organize collections of related components. The JPanel class is used to create panels.
When you create a JPanel object and add it to the JFrame such that it completely fills the content of the JFrame object, changing the color of the panel may look like the color of the content pane of the JFrame is changed. This is not so.
To change the color of the content pane of JFrame object, we call the getContentPane() method first to refer to the content pane of the the JFrame before setting the color.
Program to change the color of the content pane of the JFrame
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
package villagecoder; import java.awt.Color; import javax.swing.JFrame; /* * This program sets the color of the content pane of the JFrame to red by calling the getContentPane() */ public class ChangeContentPaneColor extends JFrame { private final int WINDOW_WIDTH=300; //width of frame private final int WINDOW_HEIGHT=150; //height of frame public ChangeContentPaneColor(){ //set title of the frame setTitle("Color of Content Pane"); //specify an action for the close button setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //set the size of the frame setSize(WINDOW_WIDTH, WINDOW_HEIGHT); //change the color of the content pane to red getContentPane().setBackground(Color.red); //make the frame visible setVisible(true); } /* * main method */ public static void main(String[] args) { //create an instance of the class new ChangeContentPaneColor(); } } |
Output
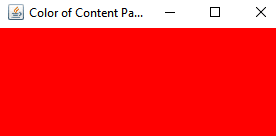
Program to change both the color of the content pane of JFrame and the panel
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
package villagecoder; import java.awt.BorderLayout; //needed for the BorderLayout class import java.awt.Color; //needed for the Color import java.awt.event.ActionEvent; //needed for the ActionEvent object import java.awt.event.ActionListener; //needed for the ActionListener interface import javax.swing.JButton; //JButton class import javax.swing.JFrame; //JFrame class import javax.swing.JLabel; //JLabel class import javax.swing.JPanel; //JPanel class /* * This program demonstrates that the panel from JPanel is different from the content pane of the JFrame. And that * the color of both can be changed independently */ public class ColorContentPaneAndPanel extends JFrame{ private JButton panelLabel; private JButton contentLabel; private JPanel panel; //the JPanel private int WINDOW_WIDTH=600; private int WINDOW_HEIGHT=150; public ColorContentPaneAndPanel(){ //set title of the frame setTitle("Change color of Content Pane and Panel"); //set the default close button setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //set the size of the frame setSize(WINDOW_WIDTH, WINDOW_HEIGHT); //set the layout of the frame setLayout(new BorderLayout()); //build panel buildPanel(); //add panel to the north region of the JFrame. Note how the north or top region turns red when //you click on the "change panel color" button add(panel,BorderLayout.NORTH); //make the frame visible setVisible(true); } /* * This method builds a panel and add buttons to it */ public void buildPanel(){ //create panel panel=new JPanel(); //create a label panelLabel =new JButton("Change panel color"); contentLabel=new JButton("Change Content Pane color"); //add action listeners to the buttons\ contentLabel.addActionListener(new ButtonListener()); panelLabel.addActionListener(new ButtonListener()); //add buttons to panel panel.add(contentLabel); panel.add(panelLabel); } /* * Private inner class to handle action events from clicking a button */ private class ButtonListener implements ActionListener{ public void actionPerformed(ActionEvent e){ if(e.getSource()==contentLabel){ getContentPane().setBackground(Color.black); } else if(e.getSource()==panelLabel){ panel.setBackground(Color.red); } } } /* * The main method */ public static void main(String[] args) { //create instance of the class new ColorContentPaneAndPanel(); } } |
Output
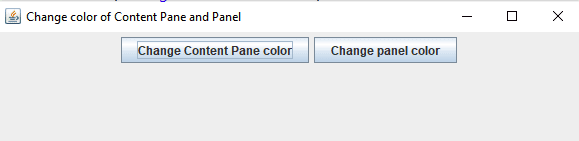
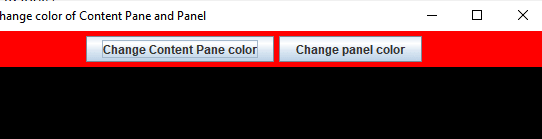
Recent Comments