Inheritance in Java – What is it?
Inheritance in Java is a process where by one class acquires the fields and methods of another class.
nanadwumor
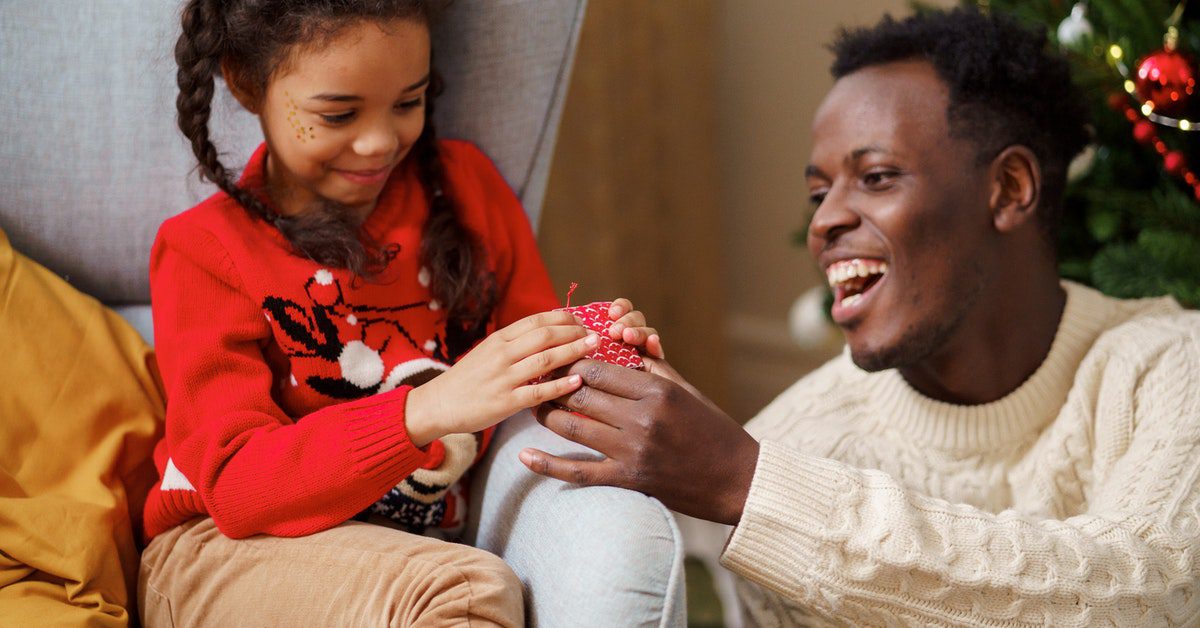
Inheritance in Java is a process where by one class acquires the fields and methods of another class.
Inheritance is one of the pillars of object-oriented programming(OOP). The others are Abstraction, Polymorphism, and Encapsulation.
The purpose of inheritance in OOP is to make a written code reusable such that a new class has to write only the unique features and rest of the common fields and methods can be inherited from the older class.
Join other Subscribers on our YouTube channel and enjoy daily pogramming tutorials.
The Superclass and subclass
If we write class A and later decide to add some functions to class A, there’s no need to rewrite the entire class from scratch if we are just tweaking the functionality of class A.
We can create a new class B with the new features, then let class B inherit the old features of class A instead of rewriting those old features and adding to class B.
In Java technical terms, we say class B extends class A. That’s, it extends the functionality of class A by adding additional code.
Recommended
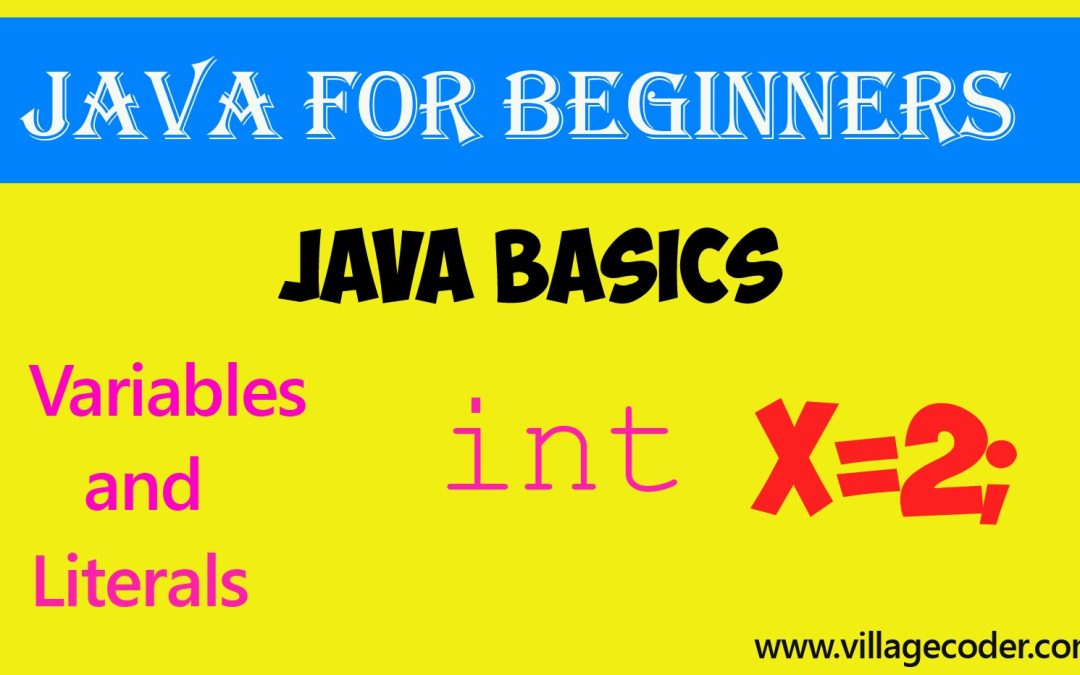
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
The class A is called the Superclass while class B is called the subclass. Alternatively, you can call class A the Parent class and class B, the child class. Some people also call class A the base class and class B, the derived class.
Syntax of Inheritance
1 2 3 |
class B extends A { } |
The “is a” relationship in inheritance
Inheritance creates what we call “is a” relationship among Classes.
Example, Let’s assume we have a class called Athlete that defines an athlete. We can also create other classes like Footballer class, BaseballPlayer class, CricketPlayer class, VolleyballPlayer class which are all different types of athletes. In other words, they are all subclasses of the superclass Athlete.
A baseball player “is a” type of athlete
A volleyball player “is a” type of athlete
A cricket player “is a” type of athlete
A footballer “is a” type of athlete
That’s, the subclass “is a” specialized type of the superclass. This is why we say inheritance always create “is a” relationship between the superclass and the subclass.
Example, in the below program, StudentExam class is used to grade students. A new class called EntranceExam will extend StudentExam in subsequent code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/** * This class grades students based on their score * * */ public class StudentExam { private double score; public void setScore(double s) { score=s; } public double getScore() { return score; } public char getGrade(){ char grade; if(score >= 90) grade='A'; else if(score >= 80) grade='B'; else if(score >= 70) grade='C'; else if(score >= 60) grade='D'; else if(score >= 50) grade='E'; else grade='F'; return grade; } } |
Program demonstrates use of the StudentExam class to grade a student
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
import javax.swing.JOptionPane; //needed for the JOptionPane class public class StudentExamDemo { public static void main(String[] args) { String input; //to input double studentScore; //to hold student's test score //Create a StudentExam object. StudentExam studentGrade=new StudentExam(); //Get student's test score input=JOptionPane.showInputDialog("Enter student's test score: "); //convert Student's test score from String to a double studentScore=Double.parseDouble(input); //Pass the score to the StudentExam object using its setScore method studentGrade.setScore(studentScore); //Display the grade of the student based on the mark scored JOptionPane.showMessageDialog(null, "Student's grade is: " + studentGrade.getGrade()); //Exit program and restore resources System.exit(0); } } |
A new class called EntranceExam extends StudentExam
Let’s assume that after using StudentExam class for sometime, we decide to organize another exam called entrance exam for students. Let’s write a class called EntranceExam to grade the new exam.
We decide that we’ll take the number of questions students answer, the number of questions they get correct or miss and points they gain into consideration.
After all these consideration, we’ll still grade the student with a letter grade so instead of adding that functionality to our new class Called EntranceExam, we decide to inherit the code we already have in the StudentExam class. That’s, we reuse that code.
To do that, we let our new class EntranceExam extend StudentExam class. This gives EntranceExam the opportunity to inherit all the members of StudentExam class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
/** * This class determines the grade of a student by using the total number of questions student * answered, total correct questions and points obtained by each correct answer. * The class extends the superclass StudentExam * * */ public class EntranceExam extends StudentExam { private int totalQuestions; //the total questions student has to answer private double pointPerQuestion; //the point acquired for each correct answer private int totalCorrect; //the total correct answers /** * The constructor sets the total number of questions student * has to answer, and the number of questions student got * correct. * @param questions The total number of questions * @param correct The number of questions answered correctly */ public EntranceExam(int questions, int correct) { double examScore; //to hold a test score //set the totalQuestions and totalCorrect fields totalQuestions=questions; totalCorrect=correct; /*calculate the point for each correct answer. Note that we want exam marked out of 100. That is if (total) questions ==> 100, then 1 question will be 100.0/questions. This is basic math, not java. We used 100.0 because we want final result to be double so that it will math the pointPerQuestion variable which is a double. Note that there will be an implicit cast from int to double even if we used 100.*/ pointPerQuestion=100.0/questions; /*This class has inherited a field called score which belongs to the superclass StudentExam * We want to set that field but before we do that, we have to find the score the student had. * Note that we weren't given the score unlike in previous program so we have to calculate the * score the student had using the number of questions he had correct, and by knowing the point * obtained from a correct answer, we can calculate the student's total score and set that superclass * field accordingly */ examScore=correct * pointPerQuestion; /*The score the student obtains is stored in the variable examScore. We can now pass the value * in this variable to the superclass score variable. Note that because the superclass setScore() * method is inherited by this class, we have call it just like any method of this class. The setScore() * method sets the value of the variable score in the superclass. */ setScore(examScore); } /*Now, we define the native methods of this subclas */ /** * The getPointPerQuestion method returns the number of points each correct answer * gives. * @return The value of a correct answer to a question */ public double getPointPerQuestion() { return pointPerQuestion; } /** * The getTotalCorrectQuestions method returns the total number of questions * student got correct. * @return The total correct answers student had */ public int getTotalCorrectQuestions() { return totalCorrect; } /** * The getTotalQuestions method returns the total number of questions * @return The total number of questions */ public int getTotalQuestions() { return totalQuestions; } } |
The ‘extends’ keyword
We see the word extends used by the EntranceExam class. This means EntranceExam class inherits all the members (fields and methods) of the StudentExam class.
Private members in inheritance
In Java, we have access modifiers. These are private, public, protected and default.
When EntranceExam class extends StudentExam class, EntranceExam class inherits all members of the StudentExam class and can access all except members that are declared private to the StudentExam class.
Thus, EntranceExam class cannot access the private field called score, inherited from StudentExam class. To access this private field, EntranceExam objects will have to use the public method called getScore() which has direct access to this private field because it is part of the StudentExam class.
Fields and Methods native and those inherited
Let’s take a closer look at the EntranceExam class now. What fields does it have now and which of them can it access?
What methods does it have now and which of them can it access?
Fields of the EntranceExam class
int TotalQuest – native to EntranceExam class
double SinglePoint – native to EntranceExam
int TotalCorrect – native to EntranceExam
Methods of the EntranceExam class
Constructor – native to EntranceExam class
getPointPerQuestion – native to EntranceExam
getTotalCorrect – native to EntranceExam
setScore – inherited from StudentExam
getScore – inherited from StudentExam
setGrade – inherited from StudentExam
The private members of the superclass
Technically, a subclass inherits all the members of the Superclass. That’s all the fields and methods.
But the private members of a superclass are literally private to the Superclass. That’s, the subclass cannot access them like the others although it can still access these private fields through a public method that can access the private field.
This means, the StudentExam class private field called score cannot be inherited by any object of the subclass. However, the public method called getScore() returns the score so it can be accessed through the method.
The other member of StudentExam that cannot be inherited by the EntranceExam subclass is the constructor of the StudentExam class. It makes a lot of sense that a superclass’s constructor cannot be inherited by its subclass though the constructor is itself a type of method.
The sole purpose of a constructor is to construct. That’s, to construct objects of its class.
So, the question is, of what importance is a constructor of a Superclass to a subclass if the constructor cannot be used by the subclass to create an object of itself?
Besides, every subclass has a constructor of its own which is sufficient to create objects of its kind.
Program demonstrates EntranceExam class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/** * This program demonstrates the EntranceExam class. This class extends the StudentExam class */ import javax.swing.JOptionPane; public class EntranceExamDemo { public static void main(String[] args) { String input; //to hold input int numQuestions; //number of questions int numCorrect; //number of correct answers //Get the total number of questions student has to answer input=JOptionPane.showInputDialog("Enter total number of questions in the Entrance exam: "); //convert input from String to int numQuestions=Integer.parseInt(input); //Get the total number of answers student had correct. input=JOptionPane.showInputDialog("Enter total number of correct answers: "); //convert input from String to int numCorrect=Integer.parseInt(input); //Create EntranceExam object EntranceExam exam=new EntranceExam(numQuestions, numCorrect); //Display the test results JOptionPane.showMessageDialog(null, "Each correct answer earns the student " + exam.getPointPerQuestion() + " points.\nStudent's score is " + exam.getScore() + ".\nStudent's grade is " + exam.getGrade()); //Exit program System.exit(0); } } |
Inheritance does not work the opposite way
A subclass extends the functionality of a Superclass. In other words, a subclass inherits the fields and members of a Superclass.
However, a Superclass cannot inherit or access any of the members of a subclass.
A real life metaphor of inheritance
Inheritance in Java is quite similar to inheritance in real life. It’s the norm for a child to inherit his parents assets but the opposite rarely happens.
An object of StudentExam cannot call these methods : getPoint, getTotalCorrect. This is because they belong to a subclass. A Superclass cannot inherit from a subclass.
The Superclass can call only its methods and fields.
So in summary, a subclass inherits all members of Superclass that aren’t private. However, a Superclass cannot inherit any member of the subclass.
You May Also Like…
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point number of 7 digits of...
Clearly explained and very concise