Java – What are Default methods in interface?
A default method is static and not abstract. It’s static means it belongs to the interface itself.
nanadwumor
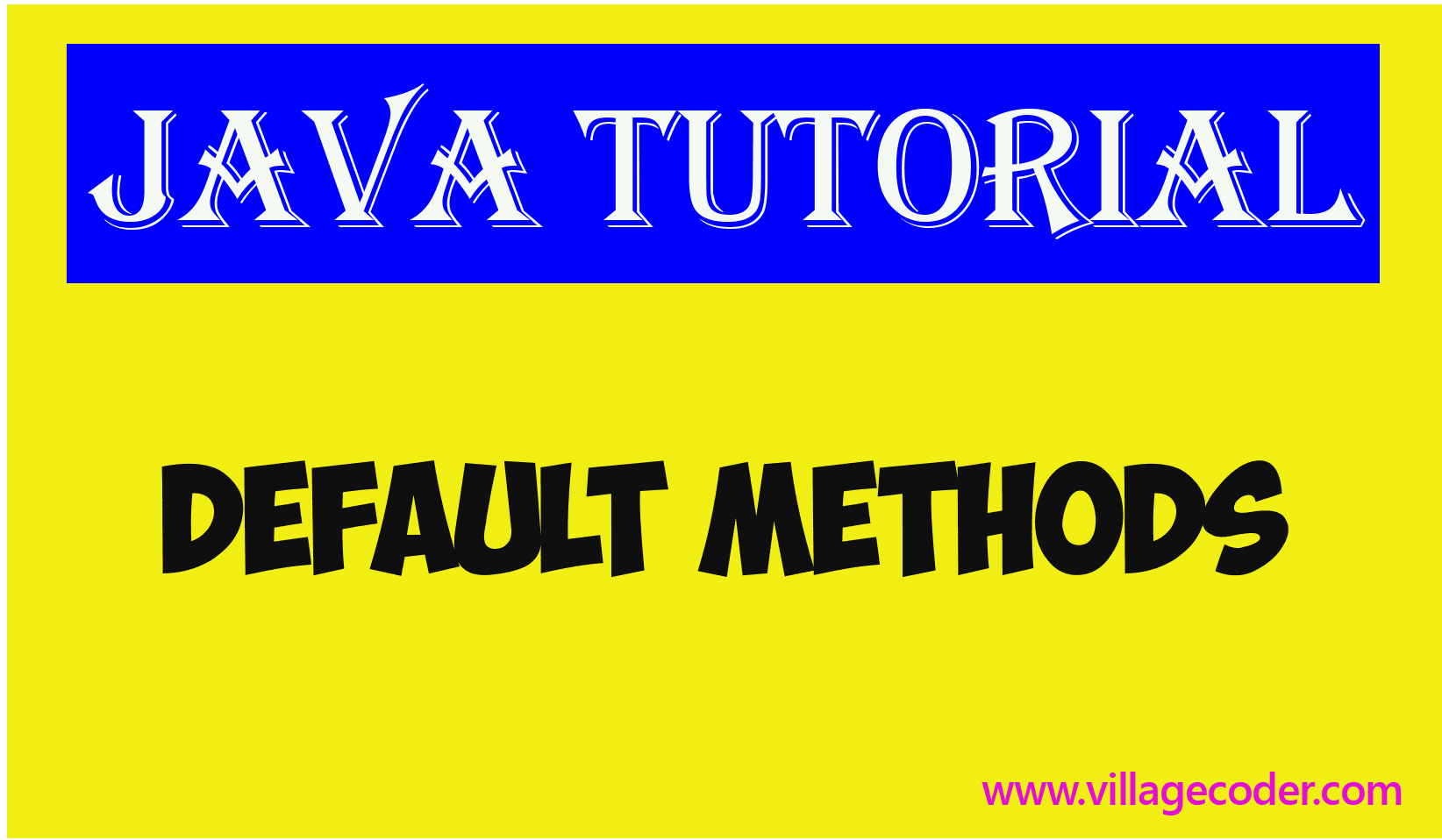
- In Java 8, default methods were introduced.
- default method is non-abstract and static method of an interface
- when a class implements an interface, it overrides its methods and provides implementation for it
RECOMMENDED ARTICLES
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures. In Java, a variable is a container used to store...
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal number. The double data type is a floating point number. It is double precision data type The range is 4.9406564584124654 x...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These methods are part of the Java API. The print and println methods are used to print string of characters onto the console The print...
A default method is a non-abstract, static method of an interface with implementation. That’s a default method is not abstract.
It belongs to the interface itself, not an instance of it (an interface cannot be instantiated) and has a body of code that can be executed by any implementing class.
Note that, when a class implements an interface, it overrides its methods and provides implementation for it. However, because a default method has implementation already, implementing classes normally don’t override them although they can.
Join Other Subscribers on Our YouTube Channel and Don’t Miss a thing!
Methods of interface were formerly all abstract methods. However, in Java 8, default methods were introduced.
A default method is static and not abstract. It’s static means it belongs to the interface itself.
Why was default method introduced in Java 8?
If an interface is implemented by a class, the class must override all of the interface’s methods and provides implementation for it.
Suppose you create an interface which is implemented by a class today. And in the future, you decide to add new features to the interface. The moment you add any new method to the interface, your classes that implement this interface must provide methods to override them.
Assume this interface is implemented by thousands of classes. Then you’d have to provide implementation for the new interface method in all the thousands of classes. That would be a nightmare. Isn’t it?
And if you fail to implement this new method in all the classes, the code will break down. Introducing default methods prevented this code breakdown because there will be no need to provide implement for a default method.
Instead of adding another abstract method that would require implementation from all the classes that implemented the interface, it will be efficient to add a default method because that won’t call for implementation from the thousand classes that implement the interface.
Program demonstrates default method
The interface is called Phone. It has two methods which are call() and message(). These methods are all abstract without any implementation.
Let’s look at two classes that implement the Phone interface. These are AndroidPhone and iPhone.
AndroidPhone class
1 2 3 4 5 6 7 8 |
/* * This class will implement the Phone interface */ public class AndroidPhone { public void model() { System.out.println("Android Phone"); } } |
Iphone class
1 2 3 4 5 6 7 8 |
/* * This class will implement the Phone interface */ public class Iphone { public void model() { System.out.println("iPhone"); } } |
Now, AndroidPhone class and iPhone class will implement the Phone interface.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
/* * The AndroidPhone class implements the Phone interface */ public class AndroidPhone implements Phone{ public void model() { System.out.println("Android Phone"); } public void call() { System.out.println ("Make a call"); } public void message() { System.out.println ("Send a text message"); } } |
Now, suppose years later, I realize that my classes – AndroidPhone and iPhone and any other class that provides similar function must be able to play computer game. Let’s call the method that ensures this function, playGame().
To ensure that all classes obey this new function, I have to add the new method to my Phone interface because this will force all classes to implement this new function.
There’s only one problem. The moment I add this new method to my interface, it’ll break my code written in the past. There will be an error unless I manually go through all the many classes that implement the Phone interface and implement this playGame() method in it. Imagine if I had about 1,000+ classes that implement the Phone interface. Horrific, isn’t it?
To ensure that I get this playGame() method or function in my code without breaking it or without having to go into each of the class one by one to implement it, I add a default method to the interface instead of an abstract method.
So, you see – a default method was introduced in Java 8 to solve the case of backward compatibility. Now let’s look at how this can be done.
Program demonstrates default method introduced in an interface to achieve a new functionality without breaking the code
1 2 3 4 5 6 7 8 |
interface Phone { void call(); void message(); default void playGame() { System.out.println("play game"); } } |
Now, let’s look at the full program.
AndroidPhone and iPhone class implement the Phone interface
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
/* * The AndroidPhone class implements the Phone interface */ public class AndroidPhone implements Phone{ /* * Method displays the model of phone * This method belongs to the Iphone class */ public void model() { System.out.println("Android Phone"); } /* * This method is inherited from the Phone interface */ public void call() { System.out.println("Make Android call"); } /* * This method is inherited from the Phone interface */ public void message () { System.out.println("Send Android text message"); } //------------------------------------------------------------- /* * This is the Iphone class. This class also implements the * Phone interface */ class Iphone implements Phone { /* * Method displays the model of phone * This method belongs to the Iphone class */ public void model() { System.out.println(" iPhone"); } /* * This method is inherited from the Phone interface */ public void call() { System.out.println("Make iPhone call"); } /* * This method is inherited from the Phone interface */ public void message() { System.out.println("Send iPhone text message"); } } //----------------------------------------------------------- /* * This is the main method. This is where the program * begins to execute. */ public static void main(String[] args){ //It can be used to reference objects of any class //that implement the Phone interface Phone aPh ; //this is an interface reference. aPh=new AndroidPhone(); //aPh.model(); //calls object instance method aPh.call(); //calls overrided interface method aPh.message(); //calls overrided interface method aPh.playGame(); //calls the interface default method //Leave double space System.out.println(); System.out.println(); //---------------------------------------------------------------- //creates another Phone interface reference variable //to reference an instance of iPhone class Phone iPh; //Note that Iphone is an inner class. In other words, it is //just like a method of the AndroidPhone class //An instance of the AndroidPhone class is needed before //an instance of the Iphone class can be accessed iPh= new AndroidPhone().new Iphone(); //iPh.model(); //calls object instance method iPh.call(); //calls overrides interface method iPh.message(); //calls overrides interface method iPh.playGame(); //calls the interface default method } } |
Output
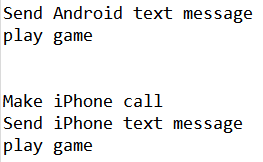
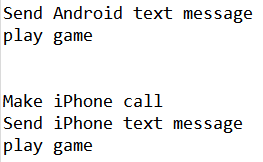
You May Also Like…
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
double data type in Java
The double data type is also a floating point number. It is called double because it has a...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the...
0 Comments