The main() method is the most important method in Java because that’s the entry point of the java program.
nanadwumor
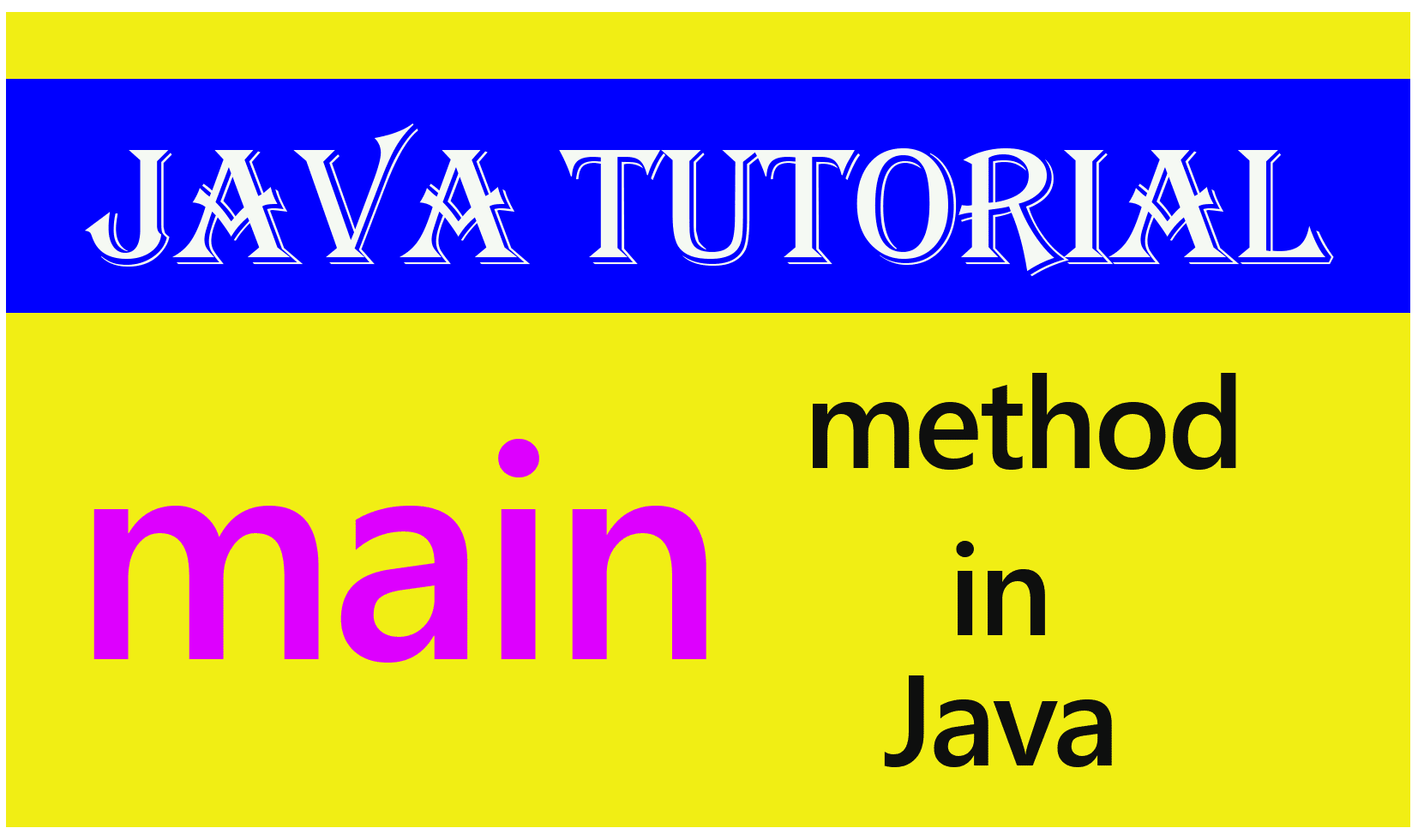
- method can be thought of as a group of one or more programming statements that collectively has a name and function
- The main() method is the entry point of the java program.
- A Java program without the main() method usually will not compile
RECOMMENDED ARTICLES
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures. In Java, a variable is a container used to store...
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal number. The double data type is a floating point number. It is double precision data type The range is 4.9406564584124654 x...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These methods are part of the Java API. The print and println methods are used to print string of characters onto the console The print...
In Java, a method can be thought of as a group of one or more programming statements that collectively has a name and function.
When you create a method, you must tell the Java compiler several things about the method.
Join Other Subscribers on Our YouTube Channel and Don’t Miss a thing!
The main() method is the most important method in Java because that’s the entry point of the java program. In other words, execution of your Java program begins from the main() method. Without the main method, the Java virtual machine (JVM) will refuse to compile your program and throw an exception or error statement.
Syntax of the main method
public static void main(String[] args){
//code here
}
or
public static void main(String args[] ){
//code here
}
Anatomy of the main method
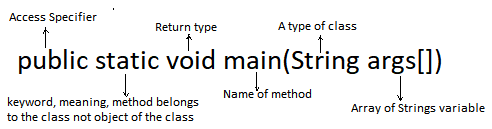
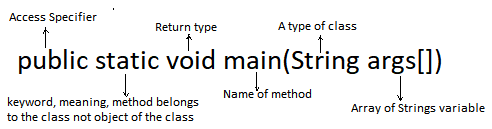
Let’s digest the main method.
main : The keyword main is the name of the method. Java is case-insensitive so uppercase letters are treated differently from lowercase letters. This means, if you type Main instead of main, the JVM will complain and throw an exception.
The main method is predefined in the JVM. Ideally, it takes the form:
public static void main ( String[] args){
//code here
}
(1) public: public is an access specifier. In other words, the keyword public specifies how the main method can be accessed. The access specifier of the main method is public.
Using public as access specifier for the main method makes it easily identifiable to the JVM. If you use any other access specifier like private, protected, or default for the main() method, the JVM will have a hard time identifying it.
As the name suggests, public means publicly available. If a method is public, any program can call it easily. Making the main program public makes it possible for the JVM to call it without problems.
Furthermore, let’s get things into perspective. When a method is declared public, it is accessible outside the class.
But when a method is declared private it implies that method is visible/accessible to only code within the class.
The main method is called by the JVM which is outside your class. The main method is in the class while the Java Runtime environment is outside your class. So by making the main method public, we make it possible for the JVM to call the method from outside the class and initiate execution of your program.
The starting point of the Java program is the main method and thus, it’s important to make it public to make it accessible to the Java compiler.
(2) static: The word static is a Java keyword. The word static means something that cannot be changed or remains unchanged.
If a method is static, it means it belongs to the class but not instances of the class (objects).
If a method is declared static, you don’t have to create an object before calling the method. In other words, static methods can be called swiftly without the need to create instances of the class (objects) first. Let’s understand static methods using an example.
Program demonstrates how static and non-static methods are called.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
import javax.swing.JOptionPane; //needed for the JOptionPane class import java.text.DecimalFormat; //needed for the DecimalFormat class public class StaticAndNonStaticMethods { public static void main(String[] args) { String input; //to hold input double miles; //to hold distance in miles double kilos; //to hold distance in kilometers //Create a DecimalFormat object to format output of distance value DecimalFormat fmt=new DecimalFormat("0.00"); //Take input from user input=JOptionPane.showInputDialog(null,"Enter the distance in kilometers: "); miles=Double.parseDouble(input); //convert the String input to double kilos=Double.parseDouble(input); //Convert from kilometers to miles JOptionPane.showMessageDialog(null, kilos + " kilometers converted to miles is: " + fmt.format(KilometersToMiles(kilos))); //Take input from user input=JOptionPane.showInputDialog(null,"Enter the distance in miles: "); //convert the String input to double miles=Double.parseDouble(input); //call the MileToKilometers. We need to create an object of StaticAndNonStatics class first //This is because the method MileToKilometers is NOT static. //Create an instance of the StaticAndNonStaticMethod StaticAndNonStaticMethods obj=new StaticAndNonStaticMethods(); JOptionPane.showMessageDialog(null, miles + " miles converted to kilometers is: " + fmt.format(obj.MilesToKilometers(miles))); } /** * The milesToKilometers method converts a * distance in miles to kilometers. * This method is NOT static so to call it, an instance of the class(object) has to be * created first * @param m The distance in miles * @return The distance in kilometers */ public double MilesToKilometers(double m){ return m*1.609; } /** * The kilometersToMiles method converts * distance in kilometers to miles. * @param kilo The distance in kilometers * @return The distance in miles */ public static double KilometersToMiles(double kilo) { return kilo/1.609; } } |
Output
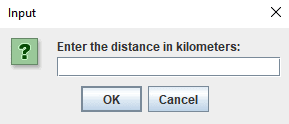
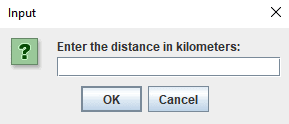
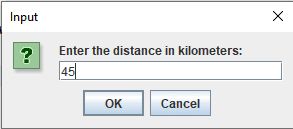
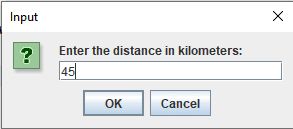
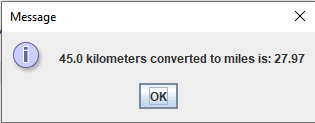
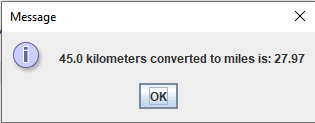
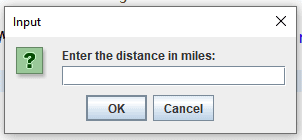
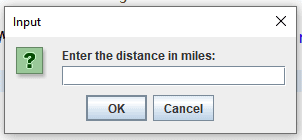
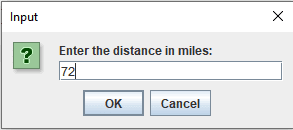
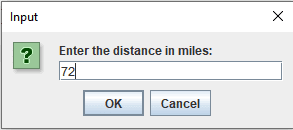
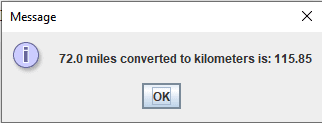
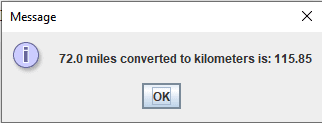
Note : In the above code, there are two methods aside the main method. These methods are the KilometersToMiles() method and MilesToKilometers() method.
The MilesToKilometers() is NOT static. This means that to call the method, an instance of the method has to be created first. This was seen in the code :
StaticAndNonStaticMethods obj=new StaticAndNonStaticMethods();
Within the statement below, we have the mile variable calling the MilesToKilometers() method.
JOptionPane.showMessageDialog(null, miles + ” miles converted to kilometers is: ” + fmt.format(obj.MilesToKilometers(miles)));
The other method, KilometersToMiles() is static. This implies that to call this method, you don’t have to create an instance of the class (that is, an object) before using the object reference to call the method. In simple terms, the KilometersToMiles() method belongs to the class instead of instance of the class.
Because Java program begins executing from the main method, it’s important that no object is required to be created before the program begins execution. Hence, the main method is always static.
If the main method is not static, then the JVM will have to first create an instance of the class before program can execute.
This can lead to problems if the class’s constructor accepts argument and the JVM becomes confused as to which constructor it should call and which parameters should the constructor accept.
(3) void: void is a Java keyword. It is a return type of the method main. In java, every method has got a return type – that’s what the method returns in the end.
As the name suggests, void means nothing. That’s the method doesn’t return any value or anything.
Java was influenced by the C-style languages like the C and C++. Interestingly, these languages have a main method that return an int value after execution. However, Java’s main method doesn’t return any value. In other words, it’s void.
If you are a C/C++ programmer, you might worry why although Java has many things in common with the C-style yet its main method is void.
(4) String args[]: String is a data type in Java. It’s a class. String[ ] args or String args[ ] means an array of String values.
The main method accepts an array of Strings. This is used to hold the command line arguments in the form of String values.
The values passed to the main() method are called arguments. These arguments are stored in the args[] array. Although the name args (short for argument) is generally used, it can be changed.
If the main() method is written without arguments
If the main method is written without arguments, the program will compile, but not run.
This is because the JVM will not recognize the main() method. The JVM always looks for the main() method with an array of string as a type parameter.
Program without the main method
1 2 3 4 5 6 7 8 |
/* * This program does not have a main method. * It won't compile */ public class NoMainMethod { System.out.print("This program has no main method."); } |
Ouput


Java before and after JDK 7
Before JDK 7, it was possible to write Java code and compile it without the main method. It was possible to write Java code under a static block without a main method and it would run fine without errors.
Program demonstrates code without main method
1 2 3 4 5 6 7 8 9 10 11 |
//This program doesn't have main method //It will run on any JDK prior to JDK 7 public class NoMainMethod { // static block static { System.out.println("This program has no main method"); } } |
Output


Using variable Strings instead of String array
A variable argument simplifies the creation of methods that need to take a variable number of arguments.
Java allows you to create a method that can take or accept varying number of arguments at differenct tmes. This argument is called a variable argument.
String…args: Using variable argument in the main method allows the method to accept zero or multiple arguments. Note that there should be exactly three dots between String and the variable, args. Anything else gives an error.
Overloading the main method
To overload a method is to create two or more methods with same name but different number and types of arguments.
The main method, like any method, can also be overloaded. That’s, we can define more than one main methods with different signature.
The signature of a method refers to the number and type of arguments it accepts.
Program demonstrates overloading of the main method
The main() method can be overloaded just like any other method. That is, we can define any number of main() methods in a class while maintaining different signatures of the methods.
Program demonstrates overloading of the main method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
//This program has overloaded main methods //First main method accepts int variable //Second main method accepts variable String arguments public class NoMainMethod { //overloaded main method public static void main(int x) { System.out.println(x); } public static void main(String args[]) { System.out.println("calling the main method"); main(6); //this calls the main method with int argument } } |
Output
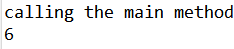
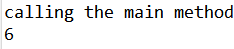
You May Also Like…
Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect...
double data type in Java
The double data type is also a floating point number. It is called double because it has a...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the...
0 Comments