Variables and Literals in Java
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures.
nanadwumor
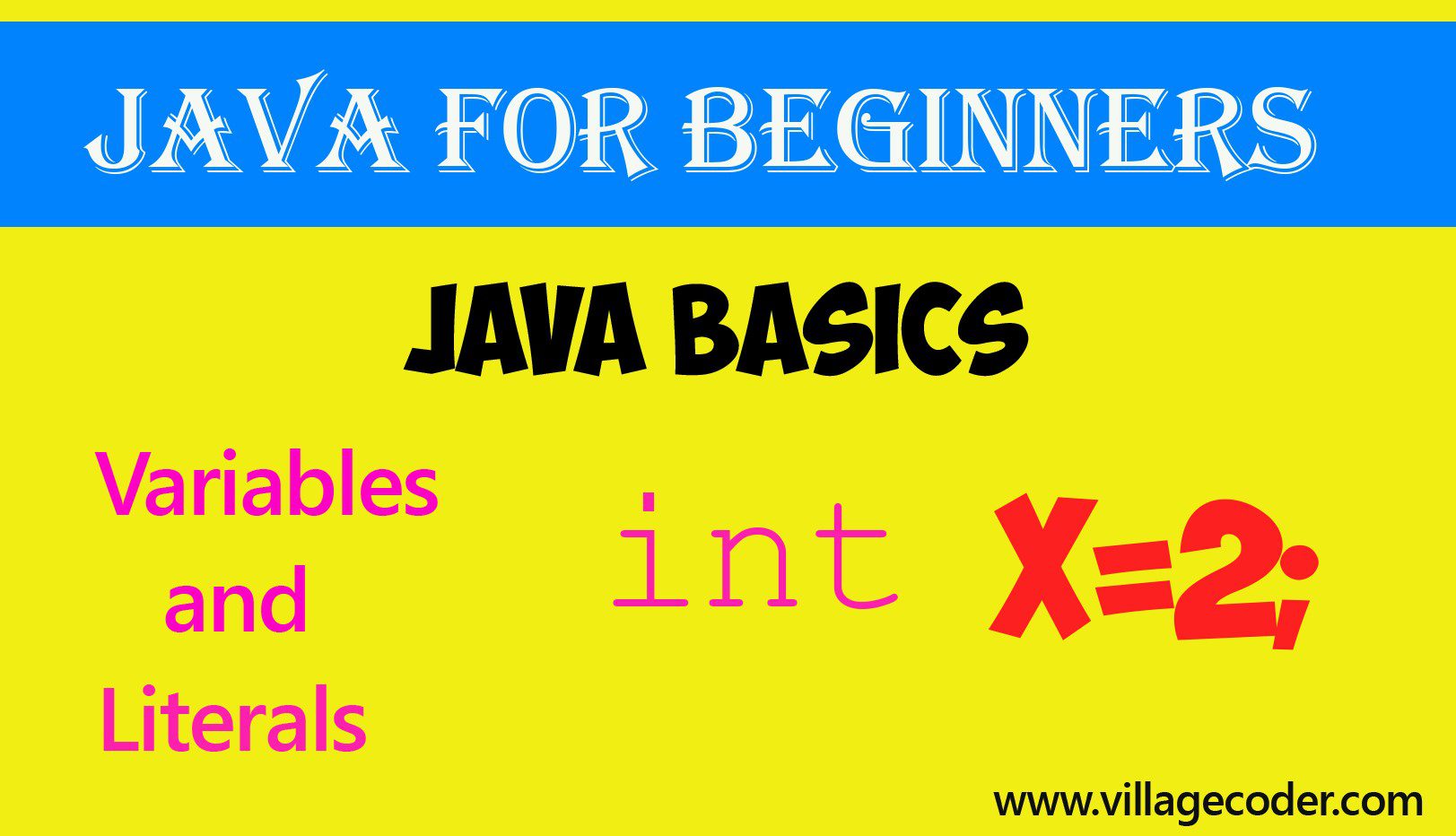
- In Java, a variable is a container used to store a data value in a computer’s memory. In other words, a variable is a storage location in a computer’s memory with a name.
-
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures.
-
To declare a variable simply means to state the data type of the variable. That’s, what type of value we want to store in the variable.
RECOMMENDED ARTICLES
double data type in Java
The double data type is also a floating point number. It is called double because it has a double-precision decimal number. The double data type is a floating point number. It is double precision data type The range is 4.9406564584124654 x...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the console. These methods are part of the Java API. The print and println methods are used to print string of characters onto the console The print...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point number of 7 digits of accuracy. The float data type is a floating point number. It has single precision data type The float type is stored in 32 bits of...
The word variable means something that is liable to change or vary. That’s something that is not constant.
In mathematics, a variable is normally denoted by an alphabet or symbol and this alphabet can hold any value.
Let’s look at some examples of how variables are presented in mathematics.
x+3=5; // x is 2
x+1=5; //x is 4
x+4=5; // x is 1
In Java, a variable is a container used to store a data value in a computer’s memory. In other words, a variable is a storage location in a computer’s memory with a name. For instance, if we type
Join Other Subscribers on Our YouTube Channel and Don’t Miss a thing!
int x=2;
this means the number 2 which is an int (integer) has been stored in the computer’s memory and a special name called x is assigned to this stored value or memory space.
Thus, if we want to refer to the number 2, we can do so by calling the letter x. The letter x is a variable and it stores the number 2 in the computer’s memory. The letter x can hold any int number.
A Java program consists of classes and a class has fields and methods. The fields are also called variables. This implies that variables are very important in a Java program. It forms part of a Java program.
Importance of variables
To understand the importance of variables in Java, let’s consider this simple narrative.
John had not seen his wife that evening. He, John, knew it was
unlike her. The night before, the two had quarreled over
petty issues. As he stood transfixed lost in thoughts,
he heard a far distant call. Was it “John” the person had shouted?
He turned swiftly and made for the door. Before he could reach for the door knob, John heard the phone ring loudly from inside the living room.
Now, imagine if the story above covered several thousands of lines and the name John ran through the entire breadth.
If in the future, the author of the story decides that the name John should be changed to another name, it would be a herculean task to have to go through the entire script and change the name one after the other.
To make it easier, he could use a variable in place of the name and set the value of this variable to John. Let’s look at what I mean.
heroCharacter had not seen his wife that evening. He, heroCharacter, knew it was
unlike her. The night before, the two had quarreled over
petty issues. As he stood transfixed lost in thoughts,
he heard a far distant call. Was it “heroCharacter” the person had shouted?
He turned swiftly and made for the door. Before he could reach for the door knob, heroCharacter heard the phone ring loudly from inside the living room.
Here, we have used a variable called heroCharacter instead of using a precise name or value. We can set and change the value of the variable as need be. That is;
heroCharacter=”John”
So to put it simply, variables help us make changes to values in our program by just changing the value of that variable once but not many times at where the value is used.
Practical Applications of variables
Variables are used in many situations. For Instance, game applications use variables to collect your name when you sign in; to store and keep track of your number of trials, successes and failures.
When you sign in to a website, variables are used to collect your name, email address and passwords. The importance of variables cannot be overemphasized.
Program demonstrates variable declaration, assignment and initialization
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
//Program demonstrates variable declaration, assignment and initialization public class VariableDemo { public static void main(String[] args) { //Declaration double price; //price is declared as double. // Assignment price=1000.0; //price is assigned the 1000 //Initialization. This is when you combine declaration and assignment double discount=0.05; //discount is initialized. That is, it is declared //and assigned a value at the same time. In other words //it is given initial value at the same time. System.out.println("The price is $" + price); System.out.println("If we apply the " + discount + "% discount, the price is $" + (discount*price)); } } |
Output


Variable Declaration
Variable Declaration is simply stating the data type of a variable. Is the variable int, double, boolean, String, Object etc ?
In the above program, we declare a variable called price as a double at line 8.
double price; //price is declared as double
This means that the variable, price will hold values of the double data type.The double data type hold floating -point or decimal values.
Java is a statically-typed language. This means that the data type of a variable in Java should be declared or first stated before it can hold a value and subsequently be used. After declaring it, the data type does not change.
Variable Declaration ends with semi-colon
A variable declaration in Java is a complete statement and ends with a semi-colon.
double price;
An error will ensue if you don’t terminate your variable declaration with a semi-colon.
double price //ERROR! No semi-colon
Variable Assignment
After declaring the data type of a variable, it is ready to store a value in it or assign it to a value. This is called variable assignment.
line 11:
price=1000.0; //price is assigned the value 1000.0
The equal sign is called an assignment operator. On the left of the equal sign is the variable. This is price. On the right is the value. This is the 1000.0
After assignment, the value 1000.0 is stored in the memory location designated as price. This means the variable price will contain the value 1000.0.
Variable Initialization
Variable initialization is when you declare the data type of a variable and assign a value to it at the same time. That is, the initial value of the variable is set at a go.
Line 14:
double discount=0.05; //discount is initialized
At line 14, a variable called discount is declared as a double and a t the same time assigned the value 0.05. We say, the variable discount has been initialized.
NOTE: Variable declaration, assignment or initialization do not print anything onto the console.
Line 18:
System.out.println(“The price is $” + price);
This line calls the println method to print or display something on the console. It is the first line that actually prints something on the console.
The string literal “The price is $” is displayed on the console. We also see the addition sign (+) and after it we see the variable, price. The addition sign concatenates the price variable to the String literal. The result is displayed by the println method.
The result is:
The price is $1000.0
Line 19:
System.out.println(“If we apply the ” + discount + “% discout, the price is $” + (discount + price);
The string literal “If we apply the ” is concatenated with the value of the discount variable, and the String literal “% discount, the price is $” and finally, (discount * price).
Note that discount*price is evaluated before it is appended to the string. That is, 0.05 * 1000 gives 50.
String Literal
When double quotation marks are placed around a value, it becomes a string literal.
Example, “The price is $” is a String literal because of the double quotation marks around it.
A string literal is printed literally to the console just as it is. However, the value of a variable is rather displayed.
The addition sign (+) and concatenation
The addition sign is used to add two numbers in mathematics. The same is true in Java.
For Instance,
System.out.print(5+6); //this prints 11
System.out.print(4+10); //this prints 14
Aside performing arithmetic operations, the addition sign can also be used to concatenate two strings in Java.
To concatenate two strings is to put them together or append one to the other. If the plus sign is used to concatenate strings, it’s called String concatenation operator.
Concatenating two Strings
When you use the plus sign to concatenate two strings, it appends one to the other.
IMPORTANT : Make sure you add a space so that when the combined string is printed, its words are separated properly
For instance,
System.out.print(“Java is an ” + “object-oriented programming language”);
Ouput


Combining Multiple Strings
You can combine as many strings as you want using the + sign. The concatenated strings are appended to each other and become one string.
System.out.print(“There are ” + “different types ” + “of programming languages ” + “which include + ” Java, ” + “Python, ” + “C, ” + “and many more.”);
Output


Concatenating a String and a value
The plus sign can also be used to append a value to a string.
System.out.println(“The number of books is ” + 15);
Output


The number of books is 15
What happens when you concatenate two strings?
When you use the plus sign to concatenate two strings, Java uses a class called StringBuilder to append one string to the other.
For example, the statement
will be implemented behind the scenes as :
String newString=(new StringBuilder()).append(“village”).append(“coder”).toString();
In Java, String concatenation is implemented through the StringBuilder (or StringBuffer) class and its append method.
When you concatenate strings, a new string is produced from appending the second operand to the end of the first.
Importance of String concatenation
There are times that the argument you pass to the print or println method is very long such that it cannot fit one line.
To remedy this, the argument passed to the method can be broken down into smaller things and concatenated.
Let’s look at an example.
1 2 3 4 5 6 7 8 |
//Program demonstrates the use of the addition operator to concatenate Strings public class StringConcatenation { public static void main(String[] args) { System.out.print("Java is one of the programming languages" + " that every programmer" + " should learn"); } } |
Output


The String literal and Double Quotation marks
Whenever you put double quotation marks on one or more characters, it becomes a String.
This can be very confusing if numbers are put in double quotation marks. For example
int score=73; //this is an integer. Correct
int mark=”73″; // mark a String value. ERROR. String cannot be stored in int variable
Numbers are used in math calculations while strings are only meant to be displayed and read.
Program demonstrates that Strings cannot be used in calculations
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
//Program demonstrates that Strings cannot be used in mathematical calculations //Strings are appended to other values when addition operator is used in between them public class StringNumber { public static void main(String[] args) { int num1=123; //correct integer number int num2=456; //correct integer number String num3="111";//String. Cannot be used in math calculation // int num4="678";//ERROR : String cannot be stored in an int variable System.out.println(num1 + num2); //gives 579 System.out.println(num1 +num3); //gives 123111. That's 111 is appended to 123 System.out.println(num3 +num1); //gives 111123. That's 123 is appended to 111 } } |
Output


num1 and num2 are both integers. The plus operator acts as a normal addition operator and adds the two numbers. That is, 123+456 gives 579. The final answer is the Integer 579. The println method converts 579 into a string and displays it on the console.
Note that we have said that Strings are meant to be read and displayed but not for mathematical computation. The println method implicitly converts the result from int to string for display purposes. The full statement is:
System.out.println(num1 + num2); //gives 579
num1 + num3 gives 123111. The num1 is an integer but num3 is a String variable. Thus, the plus sign cannot act as an addition operator. It cannot add a String to an integer. It acts as a concatenation operator and appends the 111 to 123 giving 123111. The final result is in a String and it is displayed on the console.
You May Also Like…
double data type in Java
The double data type is also a floating point number. It is called double because it has a...
The print and println methods in Java
The print() and println() methods are Java's predefined methods used to display text output on the...
float data type in Java
It is a floating point number. It has single precision data type. It stores a floating point...
0 Comments